Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial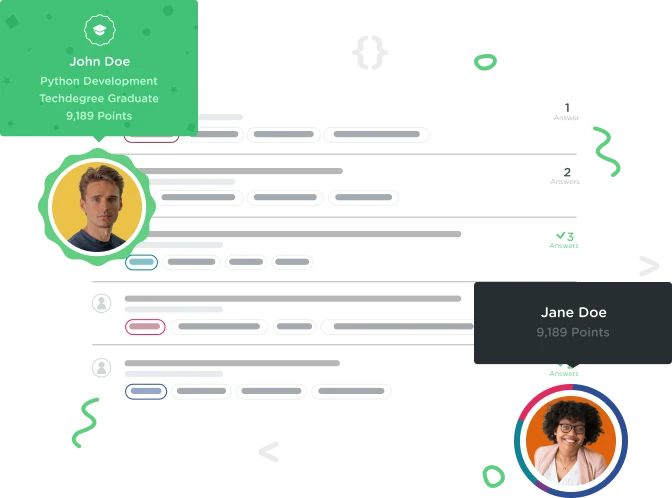
Shaun Russell
Front End Web Development Techdegree Graduate 18,510 PointsConstructor function and instance of object problems
I am having issues removing an instance of an object(assuming that's my problem). If you look at http://codepen.io/shaun0072/pen/vyJZrQ, you can play with my code, otherwise its right here:
<body>
<button id="add">Add Data</button>
<button onclick="createColorHolder(array1)">Array1</button>
<button onclick="createColorHolder(array2)">Array2</button>
</body>
var array1 = ['yellow', 'blue', 'orange', 'red', 'green'];
var array2 = ['pink', 'grey', 'tomato', 'purple', 'mustard'];
var numberToShow = 3;
function MakeSomething(array) {
$('div').remove();
for(var i = 0; i < numberToShow; i++) {
var html = '<div>';
html += array[i];
html += '</div>';
$("body").append(html);
}
document.getElementById('add').addEventListener('click', function() {
for(var i = numberToShow; i < numberToShow + 1; i++) {
var newHTML = '<div>';
newHTML += array[i];
newHTML += '</div>';
$('body').append(newHTML);
}
})
}
function createColorHolder(array) {
var makeSomething = new MakeSomething(array);
}
If you click the 'Array1' button, the first 3 colors from the variable array1 are displayed, as expected. If you click the 'Add Data' button, an additional color is added. - GOOD
Now, if you click 'Array2', the colors are replaced with 3 colors from the array2 variable. -GOOD
The problem occurs when I click 'Add Data'. I get an additional color from both my array1 and array2 variables. -BAD I only want an additional color from the array2 variable, not both!
I want a new instance of an object created by the MakeSomething() object constructor function to be created and the one before to be forgotten, but it seems to be remembering both. I am having trouble wrapping my head around why this is happening and how to fix it. I hope someone can help me understand why this is happening and what steps I can take to fix it. Thanks
1 Answer

Seth Kroger
56,413 PointsThe thing is you've already forgotten about the object as soon as you created it.
function createColorHolder(array) {
var makeSomething = new MakeSomething(array);
} // <-- makeSomething disappears here.
This isn't a great loss because it was an empty object anyway. MakeSomething() never does anything with this
to set the properties of the object. MakeSomething() looks like a straight DOM manipulation function, but not a constructor. Arguably the only object of consequence it creates is the anonymous function for clicking the Add button.
Which bring us to the actual issue. Whenever you use addEventListener() it doesn't replace the current listener, it adds an additional one. When you click one button then the next, clicking the add button will fire off both listeners. Clicking the two buttons more adds even more listeners all of which will fire off. There is a removeEventListener() method, but it requires you hold on to a reference to the listener function you can reference. A better method is to have one listener handles the add button that calls a instance method on the appropriate object. You can store the data in the object and there shouldn't be crossover.