Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial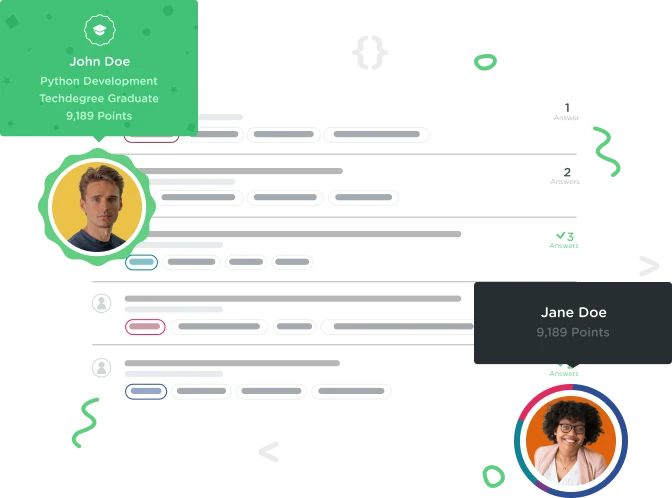

Sandy Woods
1,082 PointsConstructors
using:
private String characterName;
public PezDispenser(String name) {
characterName = name;
}
vs
private String characterName;
public PezDispenser(String characterName) {
this.characterName = characterName;
}
For the first block of code I thought a variable had to be initialized before being used as a parameter
For the second block of code, why can't we use characterName = characterName
3 Answers

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsHi Sandy,
Yes, a variable would have to be initialized and passed to the name
parameter of the constructor, but you're just writing the constructor at this point, so there won't be a variable to pass at this point. Remember that the class is a template or "cookie cutter" for creating objects of the same class. Meaning, you aren't using the constructor yet, but when you do you will have to pass an variable to the name
parameter of the constructor.
Because the class's field and the constructor parameter both share the same name, characterName, Java won't know whether you're referring to the field or parameter. That's why you need to differentiate the two by saying this.characterName = characterName;
. this
points to the object you're creating, which is a PezDispenser object, which has a characterName field.
Does that help?
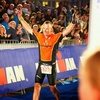
Steve Hunter
57,712 PointsHi Sandy,
As for the requirement for this
, you need to distinguish between the characterName
passed in as the parameter to the constructor and the characterName
that is the member variable of the class. The keyword this
tells the compiler that the chained-with-dot-notation variable name is part of the class, and so not a parameter.
Both your blocks of code are valid; both will do the same job.
I hope that's clear; shout if not, or if there is anything else.
Steve.

andren
28,558 PointsFor the first block of code I thought a variable had to be initialized before being used as a parameter
A parameter is a variable which is set to a value when the method is called. The value that you pass in to the method as an argument is what the parameter is set equal to. Even if a variable with a matching name already exists that doesn't actually affect the parameter at all, the parameter is its own thing and creates a new variable within the method/constructor.
For the second block of code, why can't we use characterName = characterName
The characterName
variable refers to the parameter, so if you use the code in your example you are telling Java to "Set the parameter characterName
equal to the parameter characterName
" which is pretty useless. By using the this
keyword you tell Java that you are referencing a variable that belongs to the class itself. Not a variable or parameter that is defined within the method/constructor. So this.characterName = characterName
tells Java "Set the field variable characterName
equal to the parameter characterName
" which is a lot more useful.
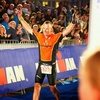
Steve Hunter
57,712 PointsLose the double-equals ...

andren
28,558 PointsOops, good catch. That's what happens when you type an answer a bit to quickly .
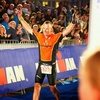