Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial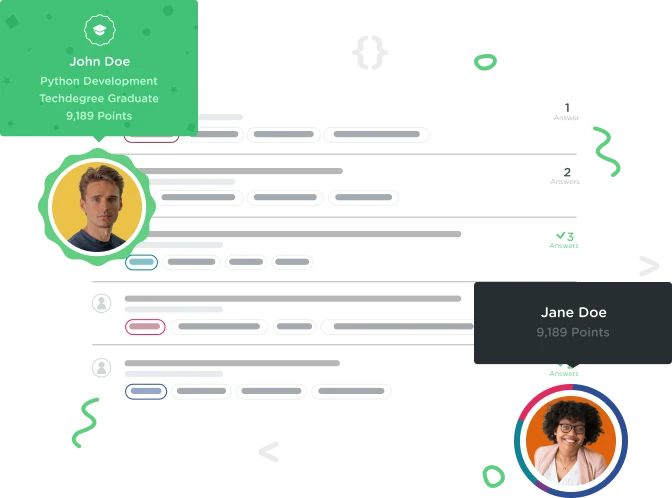

Sophie DeBenedetto
1,233 Pointscontains? method
I'm having trouble defining the contains? method to check if the todo_list contains a certain element. So far I've tried it a few different ways. Below is my latest attempt. I've also tried iterating through the array and setting a contains variable to return true if the index element == the named argument. Neither way seems to be working. Any suggestions would be greatly appreciated!
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
if todo_items.include?(name)
return true
else
return false
end
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end
2 Answers

Stone Preston
42,016 Pointshmm your code looks correct.
there is an even simpler way since the include? method itself returns a boolean is just to throw out the if/else and just call include?:
def contains?(name)
todo_items.include?(name)
end
the above code should return true if the the array contains the parameter name, and false otherwise
however that fails as well and im not too sure why its not passing. the error says its not returning a boolean but it has to be. tried using an explicit return as well and that didnt work either.
Jason Seifer any ideas whats going on?

Jason Seifer
Treehouse Guest TeacherHey Sophie DeBenedetto, great question! Check out this answer for why the initial #contains?
method didn't work.
Jess Pendley
13,128 PointsJess Pendley
13,128 PointsI am having the same problem, even when using an explicit return value.
Sophie DeBenedetto
1,233 PointsSophie DeBenedetto
1,233 PointsGot it to work by copying and slightly altering code from find_index method:
Not sure why it is necessary to create contains variable and then iterate through todo_items array though...