Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial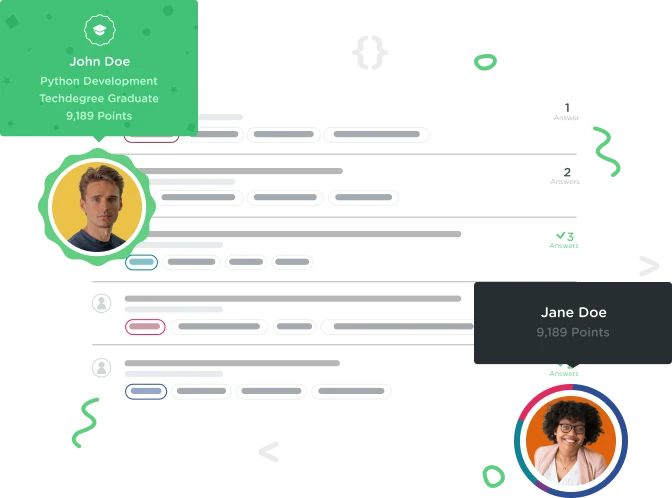
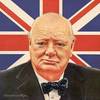
umair awan
221 PointsControl Flow-For Loops
I have no idea how control flow works. I have watched the video like 8 times and it's still confusing. I'm just not understanding the concept. Is there blog or an article describing how control flow works. Any help would be greatly appreciate it.
2 Answers
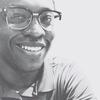
Ricardo Hill-Henry
38,442 Points@Rodrigo, great explanation, but I doubt he can understand all the terminology you're using, considering he's having trouble with loops.
To begin, a for loop, while loop, and do-while loop all essentially do the same thing. They repeat the code within their "blocks" if the code passed into it evaluates to be true. Here's an example:
for( int x = 0; x < 99; x++){
printf("Hello, can you hear me now?");
}
The for loop goes though those parenthesis like so:
- Alright, I see an integer, x, is equal to 0. Cool.
- We want to check to see if x < 99
- If x is less than 99, that expression will return what is called a boolean value. Boolean values return only two
values: true, and false. If x checks out to be less than 99, then this value is true.
- If x is less than 99, that expression will return what is called a boolean value. Boolean values return only two
- The printf statement is now ran since 0 is obviously less than 99
Now you're thinking, what about the third statement? Well, this isn't executed until after the code is run.
- Now, the third statement is executed, x++. This is the same as saying x = x + 1. Just a shortcut. So now x is
equal to the value 1. - The loop now kicks back to the top and evaluates the second statement. Now x is equal to one, so we're checking to see if 1 < 99. This is true, so the cycle goes through again.
Why isn't the first statement executed each time the loop runs, setting x = 0 continuously? Well, the for loop is actually a shorthand for the while loop. That first statement is technically treated as if it was declared outside the context of the loop. You can best see this through an example of a while loop:
int x =0;
while( x < 99){
printf("Hello, can you hear me now?");
x++;
}
This does exactly the same thing as the for loop, but is a little less compact to write.
do-while loops differ in that the code will inside the loop will run at least once regardless of the condition being true or not.
Here's a good for loop syntax:
for( term1 /*variable declaration*/; term1 comparisonOperator term2; incrementOrDecrement){
//Code to execute
}
Rodrigo Chousal
16,009 PointsHello Umair!
The for() loop is basically three statements in one line. Within the parentheses following the βforβ, you have the initial expression, condition, and the loop expression. These are separated by semicolons. A for() loop is usually used to do a repetitive task. For example, printing all of the values in an array. The control flow goes as follows: when you first declare a for loop, you need to initialize a variable, compare this variable to a value, and finally increment this variable. This will create a cycle. This cycle will be repeated until the comparison in your for loop becomes false. Then, you return 0.
Hope this helps!