Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial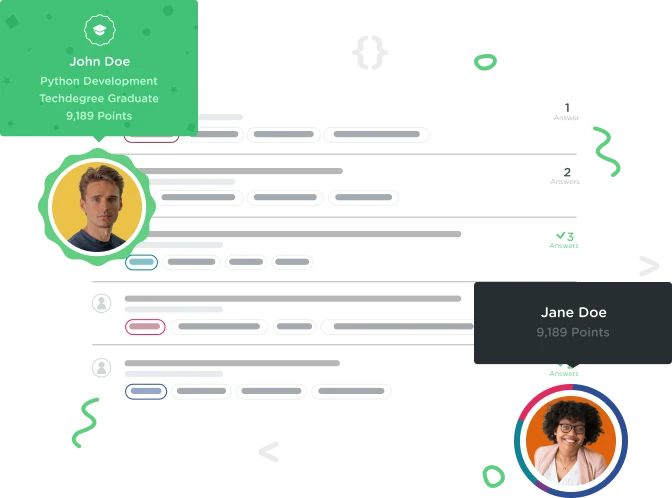
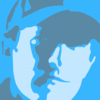
Luke Nicholls
4,536 PointsControl Structures - Fizz Buzz Extra Credit
I'm not sure if this is the right place to ask my question but I would like to know what is the correct result for the Fizz Buzz challenge. I can do it for either 3 or five resulting in the right word replacement but i'm stuck on trying to do it for both at the same time.
Thanks in advance
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Luke,
You can use the logical AND operator &&
if (number % 3 == 0 && number % 5 == 0) {
This will check that it's evenly divisible by both 3 AND 5
Or a more efficient way would be to realize that if a number is evenly divisible by both 3 and 5 then it must be evenly divisible by 15
if (number % 15 == 0) {
Whichever one you decide, it must be before the separate mod 3 and mod 5 checks.
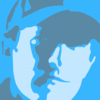
Luke Nicholls
4,536 PointsThanks Jason,
I did work it out with the following code but your way is definitely handy to know for the future.
Cheers
for (var i=100; i; i=i-1) {
var a = (i % 3);
var b = (i % 5);
if (a) {
var x = false;
}
else {
var x = true;
}
if (b) {
var y = false;
}
else {
var y = true;
}
if (x * y) {
console.log("fizzbuzz");
}
else if (x) {
console.log("fizz");
}
else if (y) {
console.log("buzz");
}
else {
console.log(i);
}
}

Michael Guren
5,195 PointsHere was my attempt:
counter = 1;
while(counter < 101){
if (counter % 15 == 0) {
console.log("fizzbuzz");
}else if(counter % 3 == 0){
console.log("fizz");
}else if(counter % 5 == 0){
console.log("buzz");
}else{
console.log(counter);
}
counter = counter + 1
}