Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial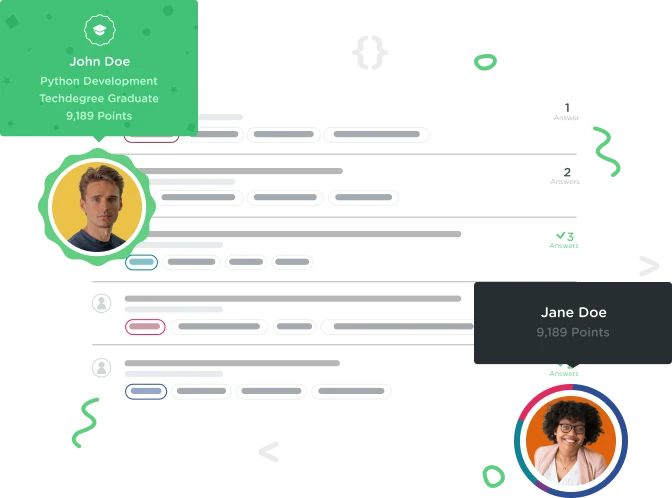

Chukwunonso Emmanuel
Courses Plus Student 3,078 Pointsconvert each int to double
using System;
class Program
{
// YOUR CODE HERE: Define a Divide method!
static void Main(string[] args)
{
// This should print "2.5".
Console.WriteLine(Divide(5, 2));
// This should print "3.3333333333..."
// (Or a value close to that, since it can't be
// infinitely precise.)
Console.WriteLine(Divide(10, 3));
}
}
1 Answer
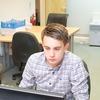
Martin Murphy
19,321 PointsJust a simple divide method but the return value needs to be converted to a double:
using System;
class Program
{
// YOUR CODE HERE: Define a Divide method!
static double Divide(int first, int second)
{
return (double) first / second;
}
static void Main(string[] args)
{
// This should print "2.5".
Console.WriteLine(Divide(5, 2));
// This should print "3.3333333333..."
// (Or a value close to that, since it can't be
// infinitely precise.)
Console.WriteLine(Divide(10, 3));
}
}