Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial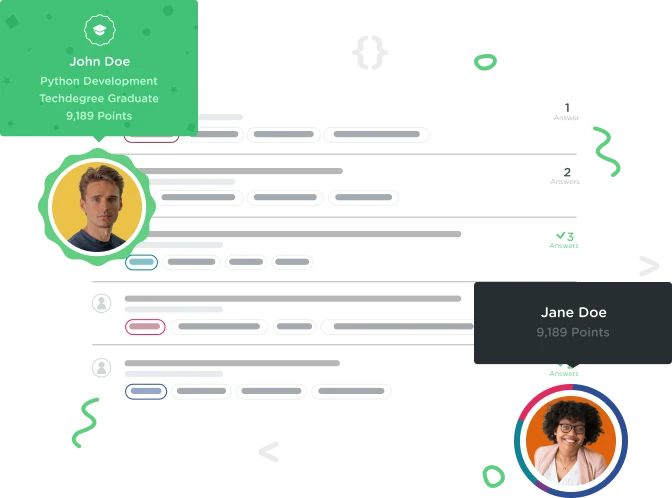

Sean Modd
4,688 PointsConvert log in to an anonymous function and pass the anonymous function directly into functionRunner.
//The app.js file originally looked like this:
function log() {
console.log("Hello World!");
}
functionRunner(log);
//My edit on the app.js file was this:
function log( callback ) {
callback();
}
log(() => console.log("Hello World!"));
functionRunner(log);
//I am confused as to where to fix this... The error I received was as follows: "There was an error with your code: TypeError: 'undefined' is not a function (evaluating 'callback()')"
function log( callback ) {
callback();
}
log(() => console.log("Hello World!"));
functionRunner(log);
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<link rel='stylesheet' href='styles.css'>
</head>
<body>
<section>
<p>Open your browser's console to see the results</p>
</section>
<script src='runner.js'></script>
<script src='app.js'></script>
</body>
</html>
function functionRunner(callback) {
callback();
}
1 Answer

Simon Coates
8,481 PointsIt seemed to accept:
functionRunner(function() {
console.log("Hello World!");
});
Sean Modd
4,688 PointsSean Modd
4,688 PointsBut that's not an anonymous function... is it??
Simon Coates
8,481 PointsSimon Coates
8,481 PointsMDN (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions) describes the following as an anonymous function and I saw similar example at w3cschools.
I think most people would immediately start to think about arrow syntax. But I tried this an it worked and it didn't seem to like my use of arrow syntax, so I guess that was what it wanted. The language i used was "It seemed to accept", which avoids committing to anything beyond passing the challenge.