Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial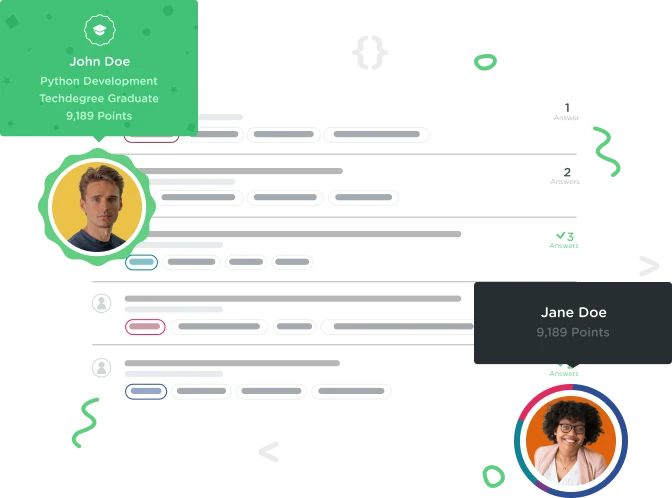

Stian Andreassen
523 PointsConvert timestamp from Firebase to readable date
I would like to convert a timestamp posted in firebase to readable data. I am able to read the timestamp, but not able to convert it, or append it to an array. This is my progress:
var orderDateHistoryArray = [String:Int]()
func getOrderDates() {
let uid = Auth.auth().currentUser!.uid
let orderDateHistoryRef = Database.database().reference().child("users/\(uid)/Orders/")
orderDateHistoryRef.observeSingleEvent(of: .value, with: { (snapshot) in
let value = snapshot.value as? NSDictionary
if let orderDate = value?["Date"] as? [Int:String] {
self.orderDateHistoryArray += Array(orderDate.values).map{ Date(timeIntervalSince1970: TimeInterval($0/1000))} // This fails. Error: "Binary operator '/' cannot be applied to operands of type 'String' and 'Int'"
print(orderDate)
}
self.tableView.reloadData()
// ...
}) { (error) in
print(error.localizedDescription)
}
}
}
The print(orderDate) statement prints correctly:
"["-LQYspEghK3KE27MlFNE": 1541421618601,
“-LQsYbhf-vl-NnRLTHhK”: 1541768379422,
“-LQYDWAKlzTrlTtO1Qiz”: 1541410526186,
“-LQsILjpNqKwLl9XBcQm”: 1541764115618]"
This is childByAutoID : timeInMilliseconds
So, I want to read out the timeInMilliseconds , convert it to a readable timestamp and append it to the orderDateHistoryArray
2 Answers
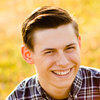
Caleb Kleveter
Treehouse Moderator 37,862 PointsFirst, you will need to convert the timestamps to Date
instances. Timestamps are usually seconds since 12:00AM, January 1st, 1970, so you would use the init(timeIntervalSince1970:)
initializer:
let timestamp: TimeInterval = 1541764115618
let date = Date(timeIntervalSince1970: timestamp)
You can then create a human readable string from that Date
instance using DateFormatter
. DateFormatter
uses strings with special placeholders to format the date's components the way you want it:
This is how you create the formatter:
let formatter = DateFormatter()
formatter.calendar = Calendar(identifier: .iso8601)
formatter.locale = Locale(identifier: "en_US_POSIX")
formatter.timeZone = TimeZone(secondsFromGMT: 0)
formatter.dateFormat = "h:mma, MMMM d, yyyy"
You can then format the date like this:
let readable = formatter.string(from: date)
If you want to mess around with date formats, I recommend this site: http://nsdateformatter.com/

Stian Andreassen
523 Points$0/1000 seems to be trying to divide a string by and int. So maybe parse it into an int first. I absolutely agree, but I have not been able to parse to int. Any suggestions?
I also don't understand what the ultimate goal is. Do you want to print out a specific date time like "1:00PM January 1st, >2018" or do you want to print out time elapsed like "4 minutes, 32 seconds and counting..."?
The goal is like you suggested:
date time like "1:00PM January 1st, >2018"
Thank you
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI'm not 100% sure, but maybe you need to convert the string to an Int first before you do the division?
$0/1000
seems to be trying to divide a string by and int. So maybe parse it into an int first.I also don't understand what the ultimate goal is. Do you want to print out a specific date time like "1:00PM January 1st, 2018" or do you want to print out time elapsed like "4 minutes, 32 seconds and counting..."?