Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial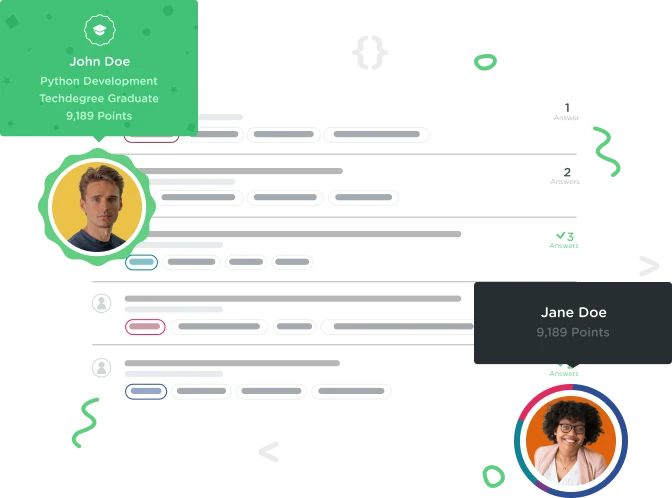
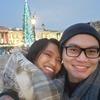
John D
7,237 PointsConverting a string to a specific type
Following is my code for the Default Function Parameters exercise. I'm trying to create a function inside my function to convert the third prompt value to undefined if I type in "undefined" for the third argument. Is it even possible to do this by using a prompt? Or do I have to hard code it via the console by calling the function explicitly?
Thanks for your help in advance!
function sayGreeting(greeting = "Wassup", name = "student") { //Default parameter acts as a fallback
return `${greeting}, ${name}`;
}
//use undefined to skip a parameter that has a default parameter in it.
const getArea = (width, length, unit = "Square Feet") => {
const area = width * length;
function convertUndefined() {
if (unit.toLowerCase() === "undefined") {
getArea(width, length, undefined);
} else {
return unit;
}
}
convertUndefined();
return `${area} ${unit}`;
}
alert(getArea(prompt("Enter a width: "), prompt("Enter a length: "), prompt("Enter a unit: ")));
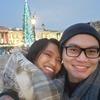
John D
7,237 PointsI'm just going by the video's tutorial with using undefined as the value to default to the given parameter of Square Feet. Leaving the 3rd prompt's answer blank still doesn't default to "Square Feet" so I tried to experiment using a nested function, but it doesn't yield the same result like explicitly passing argument of 'undefined' via the console like:
getArea(50, 50, undefined);
Output: "2500 Square Feet"
4 Answers

Simon Coates
8,377 Pointsi think the recursion (calling getArea in getArea) is probably unnecessary. If you want to use a function inside a function, then maybe something like:
const getArea = (width, length, unit = "Square Feet") => {
const area = width * length;
function convertUndefined() {
if (unit.toLowerCase() === "undefined") {
unit = "some default value";
}
}
convertUndefined();
return `${area} ${unit}`;
}
alert(getArea(prompt("Enter a width: "), prompt("Enter a length: "), prompt("Enter a unit: ")));
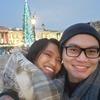
John D
7,237 PointsAh yes, this works great. I figured doubling down on calling the function within itself was redundant. I'm just messing around to see what works for me right now, I'll eventually find a more efficient way to shorten the code space. Seeing as this was a functions declaration exercise, I thought it best to use functions just to solidify the process in my head

Simon Coates
8,377 PointsI assumed it was a bit of an exercise. Your approach would have worked, I think, it was just missing a couple little things (a return statement and using the value returned). however, returning a unit and a complete answer would have made a return value unpredictable. So simplifying to have the function only change the unit seemed a good idea.

Tony Idehen
8,060 Points// setting the getArea() unit to default value, here the unit default is set // to sq m
function getArea(width, length, unit = 'sq m') {
const area = width * length;
return ${area} ${unit}
;
}
console.log(getArea(6, 4, undefined)); // this line will display result on the console: "24 sq m".

Tony Idehen
8,060 PointsThis code works for me;
const getArea = (width, length, unit ) => {
const area = width * length;
if (unit.toLowerCase() === "m" ||unit.toLowerCase() === "meter")
{
unit = 'sq m';
return ${area} ${unit}
;
} else if (unit.toLowerCase() === "ft" || unit.toLowerCase() === "feet" )
{
unit = 'sq ft';
return ${area} ${unit}
;
}
unit = 'Square Feet';
return ${area} ${unit}
;
}
alert(getArea(prompt("Enter a width: "), prompt("Enter a length: "), prompt("Enter a unit: ")));

Tony Idehen
8,060 Pointsconst getArea = (width, length, unit ) => {
const area = width * length;
if (unit.toLowerCase() === "m" ||unit.toLowerCase() === "meter") {
unit = 'sq m';
return `${area} ${unit}`;
} else if (unit.toLowerCase() === "ft" ||unit.toLowerCase() === "feet" ){
unit = 'sq ft';
return `${area} ${unit}`;
}
else
unit = 'Square Feet';
return `${area} ${unit}`;
}
alert(getArea(prompt("Enter a width: "), prompt("Enter a length: "), prompt("Enter a unit: ")));
Simon Coates
8,377 PointsSimon Coates
8,377 Pointswhy use "undefined"? if the user leaves it blank, you could do something like (unit||"default value"). If the value were left empty (ie ""), it would just use the default. And you aren't saving the value returned from your call to convertUndefined or returning the result from getArea(width, length, undefined);