Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial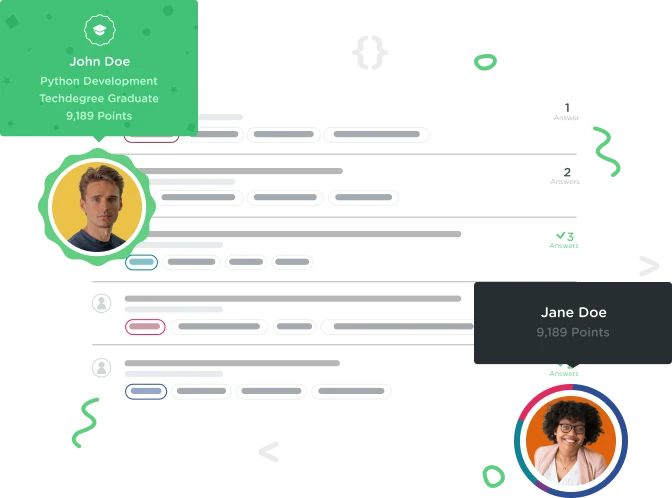

Jonathan Sylvester
915 PointsConverting Blog Reader tableview to collectionview not showing up
First off, I absolutely love this site & the friendly staff here.
I've decided to test my coding skills and convert the blog reader iOS app from inheriting from the UITableView to UICollectionView. For some reason, I can not figure out why it's showing up blank & I'm getting a couple compiler errors. Here's the code I'm using & I've provided a github link at the very bottom:
//
// TableViewController.m
// BlogReaderScratch
//
// Created by MyMac on 9/1/13.
// Copyright (c) 2013 SneakerDust. All rights reserved.
//
#import "TableViewController.h"
#import "BlogPost.h"
#import "WebViewController.h"
@interface TableViewController ()
@end
@implementation TableViewController
//- (id)initWithStyle:(UICollectionViewStyle)style
//{
// self = [super initWithStyle:style];
// if (self) {
// // Custom initialization
// }
// return self;
//}
- (void)viewDidLoad
{
[super viewDidLoad];
NSURL *blogURL = [NSURL URLWithString:@"http://blog.teamtreehouse.com/api/get_recent_summary/"];
NSData *jsonData = [NSData dataWithContentsOfURL:blogURL];
NSError *error = nil;
NSDictionary *dataDictionary = [NSJSONSerialization JSONObjectWithData:jsonData options:0 error:&error];
// NSLog(@"%@",dataDictionary);
self.blogPosts = [NSMutableArray array];
NSArray *blogPostsArray = [dataDictionary objectForKey:@"posts"];
for (NSDictionary *bpDictionary in blogPostsArray) {
BlogPost *blogPost = [BlogPost blogPostWithTitle:[bpDictionary objectForKey:@"title"]];
blogPost.author = [bpDictionary objectForKey:@"author"];
blogPost.thumbnail = [bpDictionary objectForKey:@"thumbnail"];
blogPost.date = [bpDictionary objectForKey:@"date"];
blogPost.url = [NSURL URLWithString:[bpDictionary objectForKey:@"url"]];
[self.blogPosts addObject:blogPost];
}
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView
{
// Return the number of sections.
return 1;
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return [self.blogPosts count];
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UICollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:CellIdentifier forIndexPath:indexPath];
BlogPost *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
// if ( [blogPost.thumbnail isKindOfClass:[NSString class]]) {
// NSData *imageData = [NSData dataWithContentsOfURL:blogPost.thumbnailURL];
// UIImage *image = [UIImage imageWithData:imageData];
//
// cell.imageView.image = image;
// } else {
// cell.imageView.image = [UIImage imageNamed:@"treehouse.png"];
// }
cell.textLabel.text = blogPost.title;
cell.detailTextLabel.text = [NSString stringWithFormat:@"%@ - %@",blogPost.author,[blogPost formattedDate]];
return cell;
}
- (void) prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
NSLog(@"preparing for segue: %@",segue.identifier);
if ( [segue.identifier isEqualToString:@"showBlogPost"]) {
NSIndexPath *indexPath = [[self.collectionView indexPathsForSelectedItems] lastObject];
BlogPost *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
WebViewController *wbc = (WebViewController *)segue.destinationViewController;
wbc.blogPostURL = blogPost.url;
[segue.destinationViewController setBlogPostURL:blogPost.url];
}
}
@end
github repo: https://github.com/SylJonny/blogrdr
Thank you in advance :)
1 Answer
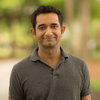
Amit Bijlani
Treehouse Guest TeacherA UICollectionViewCell
does not have the properties textLabel
or detailTextLabel
. You have to subclass it and add your own views or controls to it.