Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial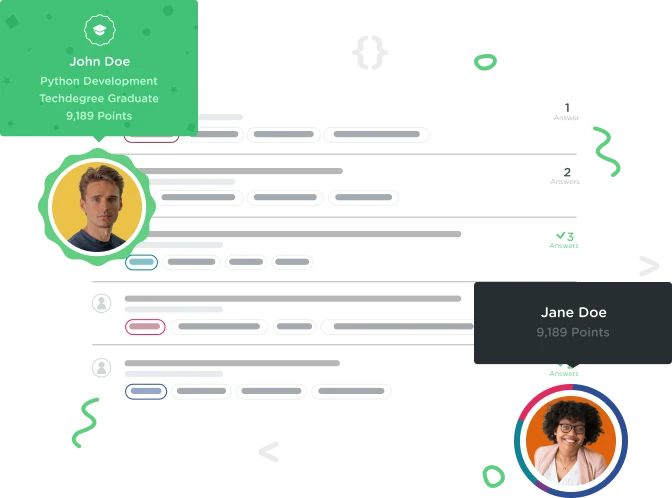
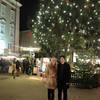
nicholas maddren
12,793 PointsCookie isn't being saved
Hi guys, just following Andrews video and got to the part where he is refreshing his page and seeing the cookie saved.
When I refresh the page it just shows me the form again instead of the variable that should be saved in the cookie.
Here is my code:
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({ extended: false }));
app.use(cookieParser());
app.set('view engine', 'pug');
app.get('/', (req, res) => {
res.render('index');
});
app.get('/cards', (req, res) => {
res.render('card', {prompt: 'Who is buried in grants tomb.', hint: "Think about who's tomb it is"});
});
app.get('/hello', (req, res) => {
res.render('hello', {name: req.cookies.username});
});
app.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.render('hello', {name: req.body.username});
});
app.listen(2828, () => {
console.log('The application is running on port 2828');
});
Any idea where I might be going wrong so far? This is really confusing me.
Thanks, Nick
1 Answer
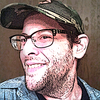
Steven Ventimiglia
27,371 PointsJust be sure you're adding it to app.get
, as opposed to app.post
...
app.get('hello', (req, res) => {
res.render('hello', {name: req.cookies.username});
});
...using app.post
to grab the info posted, and turning it into a cookie via res.cookie
.
Example:
app.get('/hello', (req, res) => {
res.render('hello', {
name: req.cookies.username
});
});
app.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.render('hello', {
name: req.body.username
});
});
- You are rendering
req.cookies.username
inapp.get
. - You are rendering
req.body.username
inapp.post
.
Nick Trabue
Courses Plus Student 12,666 PointsNick Trabue
Courses Plus Student 12,666 PointsIt looks like you're overwrite name with req.body.username. Also, it looks like the correct way to call req.cookies is
req.cookies['username']
see if that works