Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial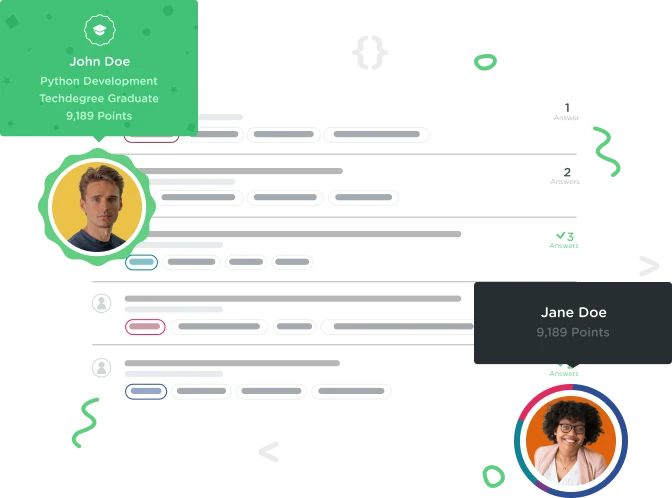
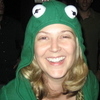
Ginny Pennekamp
31,466 PointsCopy and paste your models, views, and performer template from Workspaces into the correct files below.
Why is this code not passing?
from django.db import models
# Write your models here
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
length = models.IntegerField(default=0)
performer = models.ForeignKey("Performer")
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
class Performer(models.Model):
name = models.CharField(max_length=255)
def __str__(self):
return self.name
from django.shortcuts import get_object_or_404, render
from .models import Song, Performer
def song_list(request):
songs = Song.objects.all()
return render(request, 'songs/song_list.html', {'songs': songs})
def song_detail(request, pk):
song = get_object_or_404(Song, pk=pk)
return render(request, 'songs/song_detail.html', {'song': song})
def performer_detail(request, pk):
performer = get_object_or_404(Performer, pk=pk)
return render(request, 'songs/performer_detail.html', {'performer': performer})
{% extends 'base.html' %}
{% block title %}{{ performer.name }}{% endblock %}
{% block content %}
<h2><a href="{% url 'songs:detail' pk=performer.song.pk %}">{{ performer.song.title }}</a></h2>
{% endblock %}
5 Answers
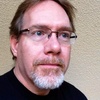
Chris Freeman
Treehouse Moderator 68,423 PointsHi Ginny, I compared your code to a known passing version and found these differences. Making these changes passes the challenge:
# in views.py file:
def performer_detail(request, pk):
performer = get_object_or_404(Performer, pk=pk)
# Add missing songs
songs = Song.objects.filter(performer=performer)
# Add songs to template context
return render(request, 'songs/performer_detail.html', {'performer': performer, 'songs': songs})
{# in performer_detail.html template, changes marked by comments such as this #}
{% extends 'base.html' %}
{% block title %}{{ performer.name }}{% endblock %}
{% block content %}
{# removed link #}
{#<h2><a href="{% url 'songs:detail' pk=performer.song.pk %}">{{ performer.song.title }}</a></h2>#}
<h2>{{ performer }}</h2>#}
{# Add loop to list songs #}
{% for song in songs %}
{{ song }}
{% endfor %}
{% endblock %}
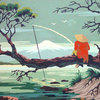
nick weber
Front End Web Development Techdegree Student 29,676 Pointsnot a fan of this challenge.

Samuel Ferree
31,722 PointsSo this whole time the challenges have been working with Articles, and writers. In the videos, Kenneth is making a learning site app, and this challenge comes out of nowhere with "Copy your songs and artists from workspaces"
What gives? I have literally no idea what the challeneg is talking about.

Cirrus Streamer
4,351 PointsThe challenge is an entirely new app, with new scenario and objects as a pretend first project. I still cant get it to pass.
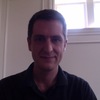
Max Hirsh
16,773 PointsIf you run the project in Workspaces and it passes all the tests, it could be a timeout error. I ran into this issue myself, and the way I solved the problem was by attempting to submit it multiple times/at different times of day. You could even try using a different browser and see if that helps. The issue seems to be that the project is fairly large and sometimes takes longer than the time limit to evaluate.

cj thompson
Courses Plus Student 3,456 PointsChris, I think I realized the error...I wasn't using Workspaces, I was working along in Django. Thanks for the response. I'll try it again in a few days...
EDIT:
I meant I was following along in PyCharm. Jeez. Long day. But I think my problem is that I need to use workspaces. For whatever reason.
Thanks again for responding so quickly.
cj thompson
Courses Plus Student 3,456 Pointscj thompson
Courses Plus Student 3,456 PointsI've tried making the same changes and can't get it to pass...
Seems like it should.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 Pointscj thompson: I suggest you create a new forum post and include your code.
nicole lumpkin
Courses Plus Student 5,328 Pointsnicole lumpkin
Courses Plus Student 5,328 PointsChris Freeman I am so confused. If views.performer_detail passes performer to performer_detail.html, how in the world are you able to pull off your for song in songs loop?
nicole lumpkin
Courses Plus Student 5,328 Pointsnicole lumpkin
Courses Plus Student 5,328 PointsWhat I really want to know Chris Freeman is why my version of performer_detail.html fails the last assertion in the last test in tests.py ?
UPDATE: Guess what ya'll! With a little help from a developer friend of mine, we figured it out! It turns out that Django was turning my greater than/less than characters('<title> by <artist>' ), to '>' and '<' respectively. I'm a noob, but he explained that those characters ('>' and '<') essentially were being escaped and transformed into the strings in bold. So when self.assertContains(resp, str(self.song)) went looking for '<I Wanna Be Sedated> by <The Ramones>', it instead found '>I Wanna Be Sedated<by>The Ramones<' BUT, if you use the filter safe it prevents this! Check out my passing performer_detail.html!!!
This also passes!!!
WHOOP WHOOP!!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 Pointsnicole lumpkin, So it looks like it's all sorted? Great work!!