Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial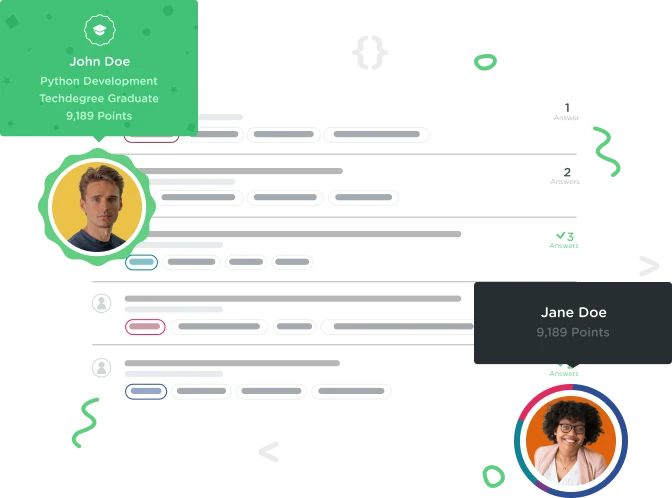

Austin Miller
5,171 PointsCorrect answers are not counting
None of my answers are counting when I run the program.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const answer1 = prompt("What is my name?");
if (answer1.toUpperCase() === "Austin") {
correct += 1;
}
const answer2 = prompt("What is my favorite color?");
if (answer2.toUpperCase() === "purple") {
correct += 1;
}
const answer3 = prompt("What is my favorite hobby?");
if (answer3.toUpperCase() === "video games") {
correct += 1;
}
const answer4 = prompt("What is my dogs name?");
if (answer4.toUpperCase() === "Kane") {
correct += 1;
}
const answer5 = prompt("Who do I look up to the most?");
if (answer5.toUpperCase() === "Dad") {
correct += 1;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correct === 5) {
rank = 'Gold';
} else if (correct >= 3) {
rank = 'Silver';
} else if (correct >= 1) {
rank = 'Bronze';
} else {
rank = 'None';
}
// 6. Output results to the <main> element
main.innerHTML = `
<h2>You got ${correct} out of 5 questions correct.</h2>
<p>Crown earned: <strong>${rank}</strong></p>`;
3 Answers

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,934 Points// the problem is in your if conditions
(answer1.toUpperCase() === "Austin") // condition is false, AUSTIN does not equal to Austin
(answer2.toUpperCase() === "purple") // condition is false, PURPLE does not equal to purple
(answer3.toUpperCase() === "video games") // condition is false, VIDEO GAMES does not equal video games
(answer4.toUpperCase() === "Kane") // condition is false, KANE does not equal Kane
(answer5.toUpperCase() === "Dad") // condition is false, DAD does not equal Dad
// in each condtion you use .toUpperCase() which converts whatever you pass to answer1, 2, 3, 4, and 5 into ALL UPPERCASES
// String comparison is case sensitive, in your case(answer1) you are comparing AUSTIN === Austin,
// since string are case sensitive this condition would be false and will not execute your correct += 1
// Solution
answer1.toLowerCase() === "austin" // for all of the answer
// make all answer#.toLowerCase() and the right handed string to all lower cases too
or
answer1.toUpperCase() === "AUSTIN" // for all of the answer
// keep the to answer#.toUpperCase, but capitalize all of the right handed strings
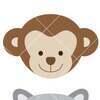
johan suh
4,157 Pointscomparison issue.
answer1 for uppercase and Austin need to be all upper case too.
const answer1 = prompt("What is my name?"); if (answer1.toUpperCase() === "Austin".toLocaleUpperCase()) { correct += 1; }
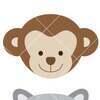
johan suh
4,157 Pointsother than that ... good looking code. .... compare to mine .... :)

jara hernández Quijada
2,475 PointsThank you very much! I was having this exact issue!
Austin Miller
5,171 PointsAustin Miller
5,171 PointsThank your for your help! I can't believe I missed that