Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial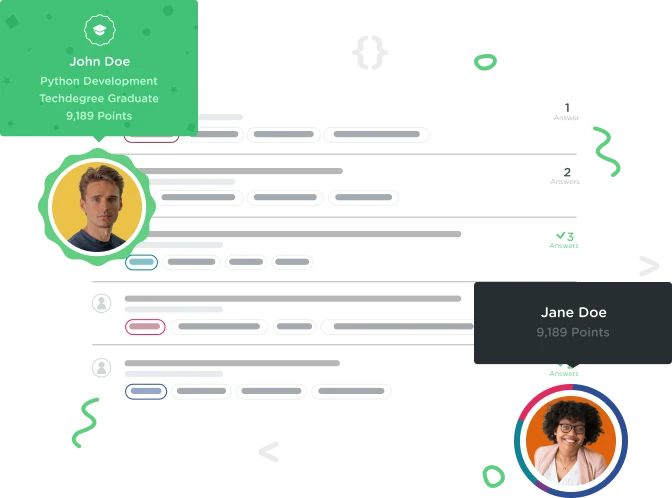

James Montgomery
5,182 PointsCorrect output "dot-dot-dot" still failing?
Code works in VSCode and displays the proper output. Not sure why it's failing here.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
intermediate_list = []
for item in self.pattern:
if item == '.':
intermediate_list.append('dot')
elif item == '-':
intermediate_list.append('dash')
for i in range(1,len(intermediate_list),1):
if i % 2 != 0:
intermediate_list.insert(i,'-')
intermediate_list.insert(-1, '-')
return ''.join(intermediate_list)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
3 Answers
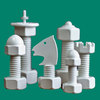
Steven Parker
229,744 PointsThe letter "S" isn't the best test since it only contains dots, but the challenge probably checks more complicated letters.
The instructions say that the output should be "dash" for every "_" (underscore), but this code seems to associate dash with hyphens instead.
Also, the mechanism for inserting separators might need revisiting, I tried encoding the morse for the number 5 (".....") and got "dot-dot-dotdot-dot".
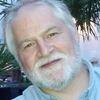
Jeff Muday
Treehouse Moderator 28,716 PointsGreat job on that. You ONLY needed to change the dash '-' to an underscore '_'
You have the makings of a very clever programmer. I see how you coded in the dash separators.
If you want, you can simplify your code further with the dash as the join-string, it should make it easier. You'll save four lines of code. See below.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
intermediate_list = []
for item in self.pattern:
if item == '.':
intermediate_list.append('dot')
elif item == '_':
intermediate_list.append('dash')
return '-'.join(intermediate_list)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)

James Montgomery
5,182 PointsThank you, both! I ended up getting rid of the hypen inserts in a list and just simplified.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
final_string = ''
for item in self.pattern:
if item == '.':
final_string += 'dot'
elif item == '_':
final_string += 'dash'
final_string += '-'
return final_string[:-1]