Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial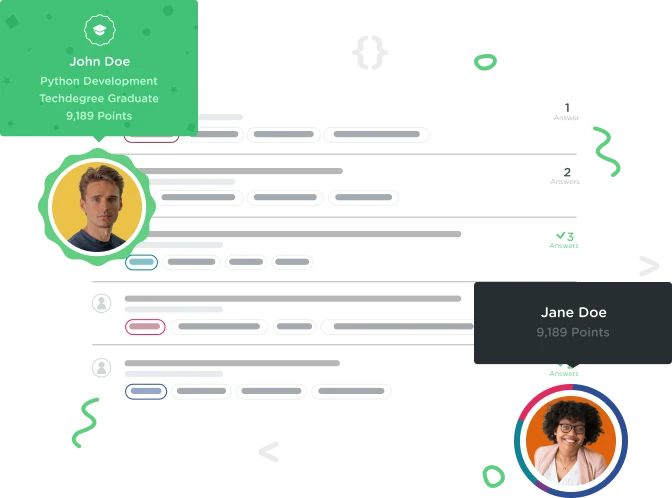

Stefan Paolini
4,679 Points"correct" variable seems to be holding proper value at end of quiz, but is displaying as "0" after each question.
The quiz seems to be working perfectly well, including giving the right crown at the end. However, for some reason the "correct" variable value doesn't seem to be updating after answering each question, displaying stubbornly at "0" the entire time. Then at the end when the conditional statements look to the "correct" value it seems to find how many questions were properly answered.
Can anyone tell me what I'm doing wrong? Thank you!
/*
Quiz Application
1. Asks >= 5 questions
2. Keeps track of # questions answered correctly
3. Provides final message telling player how many questions were answered correctly
4. Ranks player by score:
5 Gold Crown
3-4 Silver Crown
1-2 Bronze Crown
0 No Crown
*/
// GLOBAL VARIABLES //
var correct = 0;
var game = false;
var goodAnswer = 'Correct! You presently have ' + correct + ' points.'
var badAnswer = 'Wrong! You presently have ' + correct + ' points.'
// GAME START //
var opening = prompt('Welcome to the quiz game! would you like to play? Y/n');
if (opening.toUpperCase() !== 'N') {
game = true;
} else {
alert('Maybe next time!');
}
// GAME //
if (game) {
// FIVE QUESTIONS //
var q1 = prompt("1. What is the average wingspeed of an unladen swallow?")
if (q1.toUpperCase() === 'AFRICAN OR EUROPEAN') {
correct += 1;
alert(goodAnswer);
} else {
alert(badAnswer);
}
var q2 = prompt('2. Name a programming language that is also a snake.')
if (q2.toUpperCase() === 'PYTHON') {
correct += 1;
alert(goodAnswer);
} else {
alert(badAnswer);
}
var q3 = prompt('3. Name a programming language that is also a gem.')
if (q3.toUpperCase() === 'RUBY') {
correct += 1;
alert(goodAnswer);
} else {
alert(badAnswer);
}
var q4 = prompt("4. What's the capital of Italy?")
if (q4.toUpperCase() === 'ROME') {
correct += 1;
alert(goodAnswer);
} else {
alert(badAnswer);
}
var q5 = prompt("5. What's the capital of Colombia?")
if (q5.toUpperCase() === 'BOGOTA') {
correct += 1;
alert(goodAnswer);
} else {
alert(badAnswer);
}
// CROWNS //
if (correct === 0) {
alert("You suck! You get no crown!");
} else if (correct > 0 && correct < 3) {
alert("You get the bronze crown! You're OK!");
} else if (correct > 2 && correct < 5) {
alert("You get the silver crown! Pretty good!");
} else {
alert("You are the master! Gold crown!");
}
}
1 Answer

KRIS NIKOLAISEN
54,971 PointsYou have assigned goodAnswer and badAnswer at the top of your code. Those values won't change just because 'correct' did. They will keep the value at the time they were assigned. You could use functions instead and pass correct as an argument.

Stefan Paolini
4,679 PointsOK that makes sense. So even though the value of "correct" is changing later in the code, the value that is concatenated in the definition of "goodAnswer" and "badAnswer" is set?
KRIS NIKOLAISEN
54,971 PointsKRIS NIKOLAISEN
54,971 Pointsyes