Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial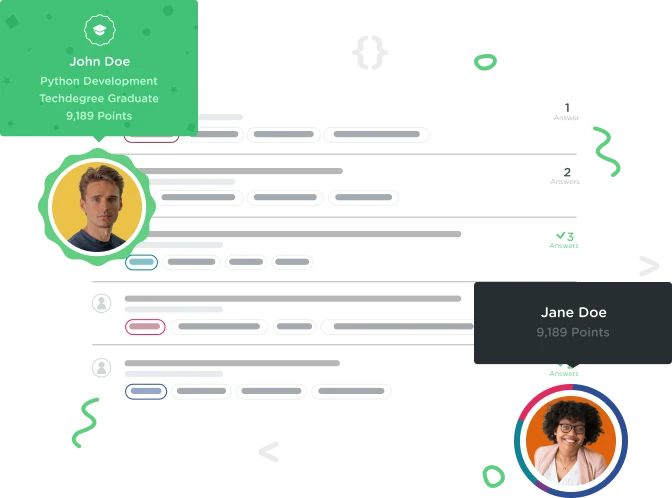

Robert Tyree
703 PointsCORS error using fetch but not Axios
Any ideas why using fetch would result in a 401 error related to CORS, but Axios works just fine?
getSearch = (userQuery) => {
fetch(`http://api.giphy.com/v1/gifs/search?q=${userQuery}api_key=dc6zaTOxFJmzC`)
.then(response => response.json())
.then(responseData => {
this.setState({ gifs: responseData.data })
})
.catch( error => {
console.log('Error of type', error);
});
}
Results in:
Failed to load https://api.giphy.com/v1/gifs/search?q=carsapi_key=dc6zaTOxFJmzC: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'null' is therefore not allowed access. The response had HTTP status code 401. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
Tried modifying fetch with
fetch(`http://api.giphy.com/v1/gifs/search?q=${userQuery}api_key=dc6zaTOxFJmzC`, {
mode: 'no-cors'
})
but still comes up with the 401 error.
Asking mainly because I recently had a code challenge for a job where they asked that you not use any third-party packages besides React, so Axios wasn't an option. Would be great to understand what fetch settings are required in this use case! Thanks in advance for any tips.
2 Answers

Seth Kroger
56,415 PointsIt happens if the API in question isn't set up to handle a CORS "pre-flight request", which is sent by the browser before your own request. The error message from Chrome is a little contradictory because mode: 'no-cors'
will only give you an anonymous response with no data, only the success or error status. If you want the data from the response you should use the option mode: 'cors'
instead.
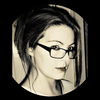
Lauren Clark
33,155 PointsJust add https:// to the call and that error will go away.