Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial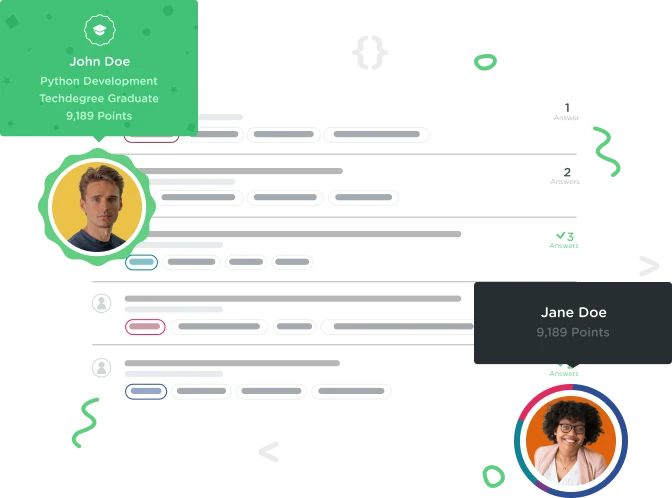
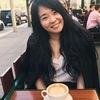
Bomi Kim
8,006 Pointscould anyone break down each line of this code?
I followed Dave's instruction, and was able to print out the list to the browser. However, I am a bit confused and having difficulties in understanding the code.
Below is the code:
var playList = [
[ 'i did it my way', 'frank sinatra'],
[ 'respect', 'aretha franklin'],
[ 'imagine', 'john lennon'],
[ 'some song', 'someone']
];
function print(message) {
document.write(message);
}
function printSongs(songs) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
Below are some of my questions:
On the 'function print(message)' and 'function printSongs(songs)', we named the parameters to 'message' and 'songs' as placeholders. Is it correct?
We didn't declare/define 'songs' parameter on any of the code before the 'function printSongs(songs) ' line. How can we make a for loop with the parameter 'songs'?
I believe I am missing something and need to understand the logic correctly before moving forward.
Thank you so much in advance!
Edited for readability by Dane E. Parchment Jr. (Moderator)
4 Answers
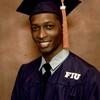
Dane Parchment
Treehouse Moderator 11,077 PointsOk, I am going to walk you through the code and then answer your questions below. Before that however, I recommend you go back and re-watch the videos covering functions in JavaScript, as all the sections after are going to assume that you understand how they work.
Now onto the code:
var playList = [
[ 'i did it my way', 'frank sinatra'],
[ 'respect', 'aretha franklin'],
[ 'imagine', 'john lennon'],
[ 'some song', 'someone']
];
This creates an array the contains arrays as its elements. In programming we call an array that contains arrays, a multi-dimensional array. These are widely used in programming specifically in programs that require vectors, or in machine learning. I recommend you make sure you understand how they work.
Basically playlist
is an array that looks like this:
Row # | Column 0 | Column 1 |
---|---|---|
0 | I did it my way | Frank Sinatra |
1 | respect | aretha franklin |
2 | imagine | john lennon |
3 | some song | someone |
As you can see you can match the row and column #s to find a specific value: (0, 0) = "I did it my way" for example. Using the same logic we can get any value in a multi-dimensional array by doing the following: playlist[0][0]
which would give us the value "I did it my way"
.
function print(message) {
document.write(message);
}
This function takes a parameter called message, and then writes whatever message is to the browser using the document.write()
method.
function printSongs(songs) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
This is the big one. printSongs(songs)
is a function that takes a parameter called songs
. In the first line of the function we establish a string called listHTML
it currently holds the string that represents the ordered list tag for html. We then create a loop that loops as many times as their are elements in the song parameter (meaning that song is either a string or some sort of list collection). We then concatenate listHTML
with another string that contains a list item tag, along with two specific variable values: songs[i][0]
and songs[i][1]
. Since we are treating song as though it is a multi-dimensional array then we can safely assume that printSongs()
accepts a multi-dimensional array as a parameter.
Now from the first part of the code we discussed, we know that we are basically printing all of the rows and columns of the multi-dimensional array.
Next we concatenate the closing ordered list tag to the listHTML
string, and then using the print()
method created earlier we print it to the html document the script is attached to. Because of the nature of document.write, and the fact that we used html tags, multi-dimensional array will be printed as an ordered list to the html page.
printSongs(playList);
This line simply calls the printSongs()
method and then gives it the parameter of the multi-dimensional array we created earlier.
Now onto your specific questions:
On the 'function print(message)' and 'function printSongs(songs)', we named the parameters to 'message' and 'songs' as placeholders. Is it correct?
Yes, parameters are basically placeholders for actual variable values, so the parameter for print()
is message
and the parameter for printSongs()
is songs
. Remember that a parameter can be anything that we put in the method that is valid javascript. So we could have written printSongs(38);
but of course that would lead to errors because a number is not what the code is expecting so when it reaches the loop within printSongs
, it will freak and error out.
We didn't declare/define 'songs' parameter on any of the code before the 'function printSongs(songs) ' line. How can we make a for loop with the parameter 'songs'?
We don't have to as it is a parameter, remember that javascript is a dynamically typed language, meaning that we don't have to specify the type of the variable that we create, the same applies for parameters. Based on how we use the parameters within the function, will give javascript's compiler and idea of what the variable we expect is. Remember, that a parameters is just a placeholder at the end of the day. If we were to define or declare song
in any way, we would change the value of the variable that we place into the function.
For example:
var num = 10;
function printNum(n) {
console.log(n);
}
printNum(num); // ====> Prints 10 to the console
var num = 10;
function printNum(n) {
n = 18;
console.log(n);
}
printNum(num); // ====> Prints 18 to the console
Do you get it now? Hopefully that helps, and again, I recommend you rewatch the video and also the videos on functions.

Thomas Nilsen
14,957 PointsWe didn't declare/define 'songs' parameter on any of the code before the 'function printSongs(songs) ' line. How can we make a for loop with the parameter 'songs'?
I'll focus on this one. It's important to understand the difference between parameter and argument in terms of functions in javascript.
//we declare the function
function foo( a, b, c ) {}; // a, b, and c are the parameters
//we call the function
foo( 1, 2, 3 ); // 1, 2, and 3 are the arguments
In the printSong-function, we've chosen to call the parameter "songs". Note: We could've called this anything. It's just a placeholder and doesn't mean a thing, although it's nice when the parameter-name explains to some extent what kind of value the function accepts.
Since we expects the "songs"-parameter to be an array, we can loop over it inside the function. We don't have error-handling inside this function, so If we called this function with a number for example:
printSongs(2);
the function would crash. because our songs-parameter got the value 2, and we would essentially be doing this inside the function:
for ( var i = 0; i < 2.length; i += 1) {
listHTML += '<li>' + 2[i][0] + ' by ' + 2[i][1] + '</li>';
}
which doesn't make any sense.
You could add error-handling like this:
function printSongs(songs) {
if(!Array.isArray(songs)) {
throw new Error("Expected array");
}
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
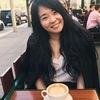
Bomi Kim
8,006 Pointsah thank you so much!
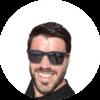
Daniel Muvdi
5,262 Pointsyes I was confuse with this as well, other way that i found to understand this is by replacing songs parameter with the real array variable name.
var playList = [
[ 'i did it my way', 'frank sinatra'],
[ 'respect', 'aretha franklin'],
[ 'imagine', 'john lennon'],
[ 'some song', 'someone']
];
function print(message) {
document.write(message);
}
function printSongs( ) {
var listHTML = '<ol>';
for ( var i = 0; i < playList .length; i += 1) {
listHTML += '<li>' + playList [i][0] + ' by ' + playList [i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
Notice that i did the same without creating a random name on the function parameter and them calling it. i just leave the parameter blank and use the array variable name instead. is the same but i think is better practice do it like the instructor did.
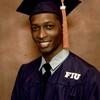
Dane Parchment
Treehouse Moderator 11,077 PointsThis may work, but you are missing something important. The whole point of the lesson was to learn and understand multi-dimensional arrays. The problem with this answer is that it actively avoids trying to implement the solution utilizing the multi-dimensional arrays.
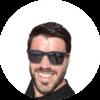
Daniel Muvdi
5,262 PointsI think you didn't understand my answer, i say this is how i finally understand what was happening on the code. i never say you should do it this way. and the reason is because in the instructor way you can re use the code in other projects.
Pd: this has nothing to do with the 2 dimensional arrays because they are used anyways in both solution. :)
Bomi Kim
8,006 PointsBomi Kim
8,006 Pointsthank you so so much dane, i now can understand the code with a logical flow.
Eddie Lehwald
2,836 PointsEddie Lehwald
2,836 PointsThanks for this fantastic explanation-I was feeling a little overwhelmed trying to understand the whole thing and going through it line made it very easy to digest.