Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial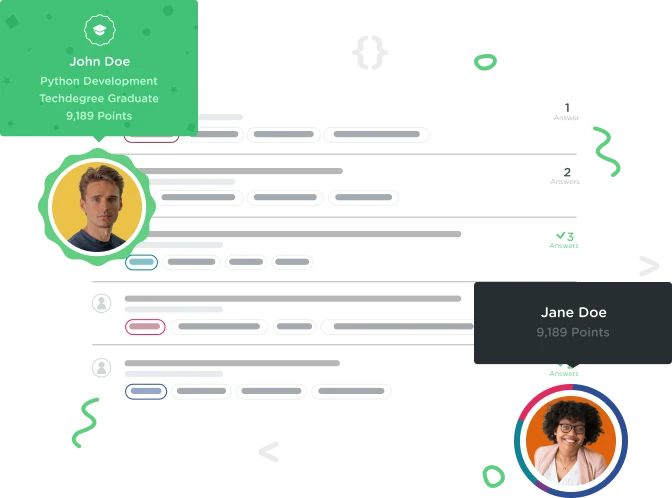

Lee Hindle
Courses Plus Student 509 PointsCould I get some feedback?
Could i get some feedback on this please? I still haven't watched the video of you explaining how you did it, just wanted to post this before I did to get some feedback, thanks!
var questionsCorrect = 0;
//Question one.
var questionOne = prompt("What is the capital of England?");
if (questionOne.toUpperCase() === "LONDON") {
document.write("<p>1: <span style=\"color: green;\">CORRECT!</span> The answer is: London.</p>");
questionsCorrect += 1;
} else {
document.write("<p>1: <span style=\"color: red;\">WRONG!</span> The answer is: London.</p>");
}
//console.log(questionsCorrect);
//Question two.
var questionTwo = prompt("What is the capital of France?");
if (questionTwo.toUpperCase() == "PARIS") {
document.write("<p>2: <span style=\"color: green;\">CORRECT!</span> The answer is: Paris.</p>");
questionsCorrect += 1;
} else {
document.write("<p>1: <span style=\"color: red;\">WRONG!</span> The answer is: Paris.</p>");
}
//console.log(questionsCorrect);
//Question three.
var questionThree = prompt("What is the capital of Italy?");
if (questionThree.toUpperCase() == "ROME") {
document.write("<p>2: <span style=\"color: green;\">CORRECT!</span> The answer is: Rome.</p>");
questionsCorrect += 1;
} else {
document.write("<p>1: <span style=\"color: red;\">WRONG!</span> The answer is: Rome.</p>");
}
//console.log(questionsCorrect);
//Question four.
var questionFour = prompt("What is the capital of America?");
if (questionFour.toUpperCase() == "WASHINGTON DC" || questionFour.toUpperCase() == "WASHINGTON" ) {
document.write("<p>2: <span style=\"color: green;\">CORRECT!</span> The answer is: Washington D.C.</p>");
questionsCorrect += 1;
} else {
document.write("<p>1: <span style=\"color: red;\">WRONG!</span> The answer is: Washington D.C.</p>");
}
//console.log(questionsCorrect);
//Question five.
var questionFive = prompt("What is the capital of Germany?");
if (questionFive.toUpperCase() == "BERLIN") {
document.write("<p>2: <span style=\"color: green;\">CORRECT!</span> The answer is: Berlin.</p>");
questionsCorrect += 1;
} else {
document.write("<p>1: <span style=\"color: red;\">WRONG!</span> The answer is: Berlin.</p>");
}
//console.log(questionsCorrect);
if (questionsCorrect === 5) {
document.write("<h2>You got " + questionsCorrect + " out of 5. You get the <span style=\"color: #FFD700;\">Gold</span> badge!</h2>");
} else if (questionsCorrect >= 3) {
document.write("<h2>You got " + questionsCorrect + " out of 5. You get the <span style=\"color: #C0C0C0;\">Silver</span> badge!</h2>");
} else if (questionsCorrect >= 1) {
document.write("<h2>You got " + questionsCorrect + " out of 5. You get the <span style=\"color: #CD7F32;\">Bronze</span> badge!</h2>");
} else {
document.write("<h2>You got " + questionsCorrect + " out of 5. You dont get a badge :(</h2>");
}
3 Answers

Michael Lambert
6,286 PointsThere are many ways to code this but all looks good and functional. One thing that did jump out at me is your method of incrementing the count for correct answers. When your only incrementing by 1, there is a shorthand method you can use:
Instead of:
questionsCorrect += 1;
you can just use:
questionsCorrect ++;

cameronkeathley
2,708 PointsI like the way you used .toUpperCase to filter out issues in different cases in the answer. I'm going to have to use that.

Curtis Simonson
UX Design Techdegree Graduate 13,791 PointsCode looks great. Very organized.
Daphne Orme
2,275 PointsDaphne Orme
2,275 Pointswhat does the +=1 or ++ mean or represent?
Michael Lambert
6,286 PointsMichael Lambert
6,286 Points+=1 & ++ do exactly the same. The both just add 1 to the current value of what is stored in the variable. If one was +=2 then the ++ wouldn't apply because ++ always add's just 1 to the current value of the variable