Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial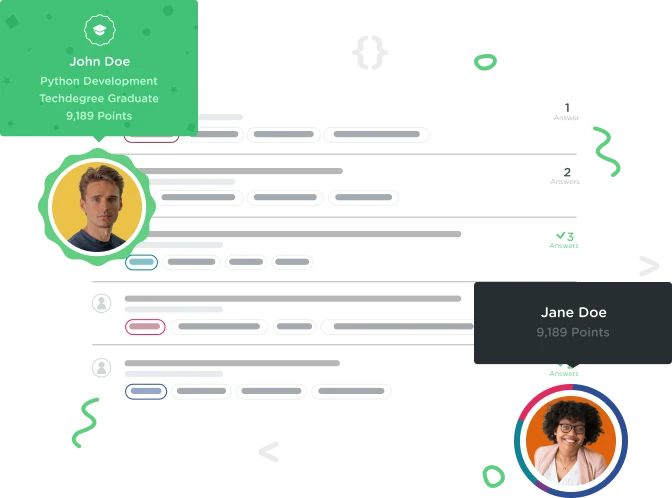
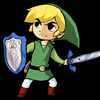
Liam Hayes
Full Stack JavaScript Techdegree Student 13,116 PointsCould somebody explain this syntax to me? nameActions[action]();
How's it going guys? Check this out, I am having trouble understanding this line of code:
nameActions[action]();
This line of code is used in the code below, at the bottom
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
const action = button.textContent;
const nameActions = {
remove: () => {
ul.removeChild(li);
},
edit: () => {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
},
save: () => {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
};
// select and run action in button's name
nameActions[action](); // <---- HERE is that line of code
});
});
So, here is the help I need with this line of code
- I understand that this is a dynamic call of some kind
- I get that this is done instead of using a dot operator
- But I'm lost otherwise with this line
- One thing that is really throwing me off is the parenthesis at the end
- Would someone by chance be able go over this line of code and give a breakdown of what is going on with it?
Thank you VERY MUCH :)
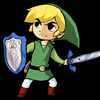
Liam Hayes
Full Stack JavaScript Techdegree Student 13,116 PointsOh, I think I get it, so basically we can dynamically call objects like this
nameActions[action];
But if we might pass in a function, we can also dynamically call objects like this
nameActions[action]();
Is that right?
2 Answers
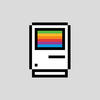
Hakim Rachidi
38,490 PointsSo basicly you got this already:
I understand that this is a dynamic call of some kind
In JavaScript you can access a property or a method through the dot-syntax like in most programming languages
var a = {foo: "bar"}
console.log(a.foo)
// -> bar
But JavaScript offers another powerful way of doing this dynamicly.
var prop = "foo";
// this works (uses the string as the property name)
console.log(a[prop]);
// -> bar
// this would search for a property named prop
console.log(a.prop);
// -> undefined
We could do the same thing for methods with the parenthesis at the end
var functionName = "f";
// this works (uses the string as the function name)
a[functionName]();
// this would search for a function named functionName
a.functionName();
//-> TypeError a.functionName is not a function
In your example the action ( the button name ) is the key to a function which is then called.
Hope this helps;
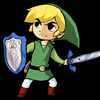
Liam Hayes
Full Stack JavaScript Techdegree Student 13,116 PointsBro, thank you very much. That helped A LOT.
I just have one quick follow up question.
Can you use this dynamic call WITH parenthesis, the same kind of dynamic call you would use for a function, to access an object and not a function?
I learned from you that we can access an object like you said, like this a[prop], but could it also be accessed like this too?
var prop = "foo";
a[prop]();
The reason I ask is because that's what I think the guy in the video did, he used the brackets plus parenthesis code on an actual object, unless I'm maybe wrong about that
It looks like he is using this code
nameActions[action]();
To access this object
const nameActions = {
remove: () => {
ul.removeChild(li);
},
edit: () => {
const span = l
}
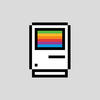
Hakim Rachidi
38,490 PointsHi Liam Hayes,
You said:
The reason I ask is because that's what I think the guy in the video did, he used the brackets plus parenthesis code on an actual object,
name : () => {
ul.removeChild(li);
},
...
is a shorthand for this:
name : function () {
ul.removeChild(li);
},
...
So these are all functions in an object.
Your example would not work because thenever you call a function you use parenthesis. Not with properties.
var prop = "foo";
a[prop]();
This would end in an TypeError : a.foo is not a function.
Hope this helps;

Siddharth Pandey
7,280 PointsCorrect me if I am wrong, but does (); just tell the browser to search for a function?
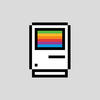
Hakim Rachidi
38,490 PointsSiddharth Pandey, the browser already searches for the memory block in which the property (in the case of the function an instruction set) resides in, when you access the property with either bracket or dot-syntax. The () just executes the instruction set of the function (the function body).
Access of function. (Browser is looking up the address of the function)
a.func
Browser is executing the instruction set which a.func
is pointing to.
a.func()

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsHey Liam Hayes . I get the same feeling when I first watched the video. Actually he is doing so much stuff in this video .
Let's go step by step -
- He made the object named
nameActions
.
-
nameActions
has three methods.
a. remove
b. edit
c. save
(A JavaScript method is a property containing a function definition) :
Note : You access an object method with the following syntax:
objectName.functionName()
or myobject["myfunction"]()
;
You can't use dot notation here , because , you have to substitute the value of action value then call the corresponding method.
- Now , suppose user clicked on
remove
button you will call the remove function like this-
nameActions[remove]();
Similary if user clicked on edit
button , you will call the edit function like this-
nameActions[edit]();
Similary if user clicked on save
button , you will call the edit function like this-
nameActions[save]();
Now , you can see the same line is repeated , what's changing is properties .
But, if you will see there is , the properties of nameActions
object are same as the textContent of the button.
So , in place of writing properties , we can extract the textContent of the button , the user clicked on , then save it into a new variable and finally call the function like this -
const action = button.textContent;
nameActions[action](); // nameActions[action] is getting the property and () is calling that function
Summary - The action will only be one of these three possible strings,
right, remove, edit, or save. In the if statement, action is being compared to each possible string.
So when a match is found, that same word will be used to run the branch.
Hope it helps :)
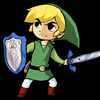
Liam Hayes
Full Stack JavaScript Techdegree Student 13,116 PointsHow's it going bro :)
I just wanted to thank you very much for you answer. It just so happens that I already had understood the part you explained, and I was having trouble understanding something else about the last line of code.
Thanks for taking the time to answer man.
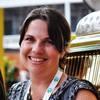
Cheryl Oliver
21,676 PointsThanks so much, I was struggling with this and you explained it so well!
Robert Strickland
Courses Plus Student 171 PointsRobert Strickland
Courses Plus Student 171 PointsThe parenthesis at the end means the line will try to execute a function if one is assigned to the object 'nameActions' at the property indicated by the value of button.textContext. If button.textContext is 'save' then the 'save' function is executed in that last line. If the value fails to match any function in the object then you will get an exception stating the value is not a function.