Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial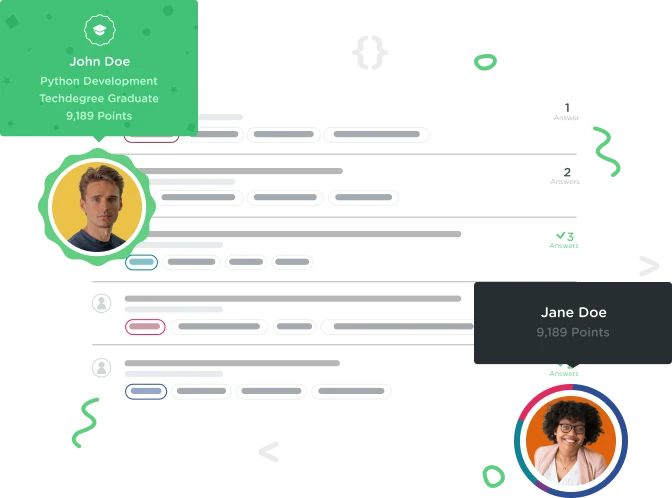
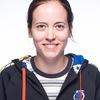
Jo Lingenfelter
Treehouse Project ReviewerCould somebody help me with the second Code Challenge? I'm having trouble with inheritance.
I would just really like to see a solution to this, so I can understand how to make it work. I can't get my Robot class to recognize the x and y properties of Point.
7 Answers
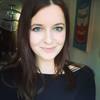
Marina Alenskaja
9,320 PointsHi Gibson
Here is the correct answer. Although the switch statement is optional - you could use if else instead, I just think the switch statements are easiest and most intuitive.
We switch on the direction that we pass into the function. And depending on the string, we want to increase or decrease the x and y coordinates of the Point class, which is stored in the subclass location. This is why we use location.x and not for example point.x
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Right": location.x += 1
case "Left": location.x -= 1
default: break
}
}
I hope this helps.
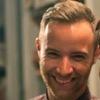
Gibson Smiley
1,923 PointsHaving trouble on this challenge too, anyone mind posting the correct code so I can see what I'm doing wrong?
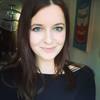
Marina Alenskaja
9,320 PointsHi! Can you paste in the code you have so far?
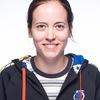
Jo Lingenfelter
Treehouse Project Reviewerclass Point { var x: Int var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine { var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine { }
Hi! Thank you!
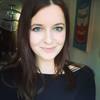
Marina Alenskaja
9,320 PointsHi again
So, you need to create a subclass of machine - which you do by writing out this code, and then pasting in the method. In the method you can then write out your if or switch statement.
class Robot: Machine {
override func move(direction: String) {
}
}
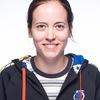
Jo Lingenfelter
Treehouse Project ReviewerFigured it out! Thanks!
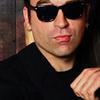
Filippo Lovotti
1,516 PointsHey,
Kinda late, but this worked for me:
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": self.location.y++
case "Down": self.location.y--
case "Left": self.location.x--
case "Right": self.location.x++
default: break
}
}
}
At first, I had some trouble calling out x and y for Robot, but I eventually figured it out.

Rich Braymiller
7,119 Pointsstill isn't working...getting this error swift_lint.swift:34:19: error: argument names for method 'move(direction:)' do not match those of overridden method 'move' override func move(direction: String) { ^ _
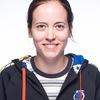
Jo Lingenfelter
Treehouse Project ReviewerCould you post your code?

Dangelot Muzeau
892 Pointshere's the Answer class Robot: Machine {
override func move(_ direction: String) { switch direction { case "Up": location.y += 1 case "Down": location.y -= 1 case "Right": location.x += 1 case "Left": location.x -= 1 default: break } } }