Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial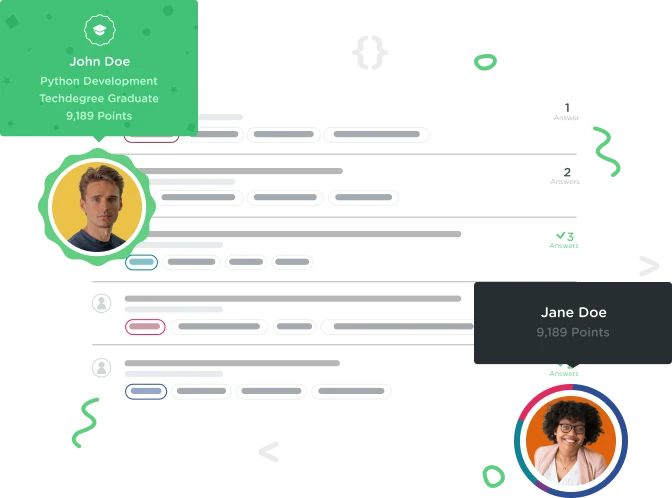

Aaron Grant
1,065 PointsCould somebody post all of there project so far. I have over 15 errors.
application_controller.rb
class ApplicationController < ActionController::Base # Prevent CSRF attacks by raising an exception. # For APIs, you may want to use :null_session instead. protect_from_forgery with: :exception end
todo_items_controller.rb
class TodoItemsController < ApplicationController before_action :find_todo_list def index end
def new @todo_list = @todo_list.todo_items.new end
def create @todo_item = @todo_list.todo_items.new(todo_item_params) if @todo_item.save flash[:success] = "Added todo list item." redirect_to todo_list_todo_items_path else flash[:error] = "There was a problem adding that todo list item." render action: :new end end
def edit @todo_item = @todo_list.todo_items.find(params[:id]) end
def update @todo_item = @todo_list.todo_items.find(params[:id]) if @todo_item.update_attributes(todo_item_params) flash[:success] = "Saved todo list item." else flash[:error] = "That todo item could not be saved." render action: :edit end end
def url_options { todo_list_id: params[:todo_list_id] }.merge(super) end
private def find_todo_list @todo_list = TodoList.find{params[:todo_list_id]} end def todo_item_params params[:todo_item].permit(:content) end
end
toto_lists_controller.rb
class TodoListsController < ApplicationController before_action :set_todo_list, only: [:show, :edit, :update, :destroy]
# GET /todo_lists # GET /todo_lists.json def index @todo_lists = TodoList.all end
# GET /todo_lists/1 # GET /todo_lists/1.json def show end
# GET /todo_lists/new def new @todo_list = TodoList.new end
# GET /todo_lists/1/edit def edit end
# POST /todo_lists # POST /todo_lists.json def create @todo_list = TodoList.new(todo_list_params)
respond_to do |format|
if @todo_list.save
format.html { redirect_to @todo_list, notice: 'Todo list was successfully created.' }
format.json { render :show, status: :created, location: @todo_list }
else
format.html { render :new }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /todo_lists/1 # PATCH/PUT /todo_lists/1.json def update respond_to do |format| if @todo_list.update(todo_list_params) format.html { redirect_to @todo_list, notice: 'Todo list was successfully updated.' } format.json { render :show, status: :ok, location: @todo_list } else format.html { render :edit } format.json { render json: @todo_list.errors, status: :unprocessable_entity } end end end
# DELETE /todo_lists/1 # DELETE /todo_lists/1.json def destroy @todo_list.destroy respond_to do |format| format.html { redirect_to todo_lists_url, notice: 'Todo list was successfully destroyed.' } format.json { head :no_content } end end
private # Use callbacks to share common setup or constraints between actions. def set_todo_list @todo_list = TodoList.find(params[:id]) end
# Never trust parameters from the scary internet, only allow the white list through.
def todo_list_params
params.require(:todo_list).permit(:title, :description)
end
end
todo_item.rb
class TodoItem < ActiveRecord::Base belongs_to :todo_list
validates :content, presence: true, length: { minimum: 2 } end
todo_list.rb
class TodoList < ActiveRecord::Base has_many :todo_items
validates :title, presence: true
validates :title, length: { minimum: 3}
validates :description, presence: true
validates :description, length: { minimum: 5}
end
application.html.erb
<!DOCTYPE html> <html> <head> <title>Odot</title> <%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track' => true %> <%= javascript_include_tag 'application', 'data-turbolinks-track' => true %> <%= csrf_meta_tags %> </head> <body>
<% flash.each do |type, message| %> <div class="alert flash <%= type %>"> <%= message %> </div> <% end %>
<%= yield %>
</body> </html>
edit.html.erb
<h1><% @todo_list.title %> - Editing Todo List Item</h1> <%= form_for [@todo_list, @todo_item] do |form| %> <%= render partial: form %> <% end %>
form.html.erb
<div id="error_explanation"> <h2><%= pluralize(@todo_item.errors.count, "error") %> prohibited this todo item from being saved:</h2>
<ul> <% @todo_item.errors.full_messages.each do |msg| %> <li><%= msg %></li> <% end %> </ul> </div> <% end %>
<%= form.label :content %> <%= form.text_field :content %>
<%= form.submit "Save" %>
index.html.erb
<h1><%= @todo_list.title %></h1>
<ul class="todo_items"> <% @todo_list.todo_items.each do |todo_item| %> <li id="<%= dom_id(todo_item) %>"> <%= todo_item.content %> <%= link_to "Edit", edit_todo_list_todo_item_path(todo_item) %> </li> <% end %> </ul>
<p> <%= link_to "New Todo Item", new_toto_list_todo_item_path %> </p>
new.html.erb
<h1><% @todo_list.title %> - Add Todo List Item</h1> <%= form_for [@todo_list, @todo_item] do |form| %> <%= render partial: form %> <% end %>
_form.html.erb
<%= form_for(@todo_list) do |f| %> <% if @todo_list.errors.any? %> <div id="error_explanation"> <h2><%= pluralize(@todo_list.errors.count, "error") %> prohibited this todo_list from being saved:</h2>
<ul>
<% @todo_list.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<div class="field"> <%= f.label :title %><br> <%= f.text_field :title %> </div> <div class="field"> <%= f.label :description %><br> <%= f.text_area :description %> </div> <div class="actions"> <%= f.submit %> </div> <% end %>
edit.html.erb
<h1>Editing todo_list</h1>
<%= render 'form' %>
<%= link_to 'Show', @todo_list %> | <%= link_to 'Back', todo_lists_path %>
index.html.erb
<h1>Listing todo_lists</h1>
<table> <thead> <tr> <th>Title</th> <th>Description</th> <th></th> </tr> </thead>
<tbody> <% @todo_lists.each do |todo_list| %> <tr id="<%= dom_id(todo_list) %>"> <td><%= todo_list.title %></td> <td><%= todo_list.description %></td> <%= link_to "List Items", todo_list_todo_items_path(todo_list) %> <%= link_to 'Show', todo_list %> <%= link_to 'Edit', edit_todo_list_path(todo_list) %> <%= link_to 'Destroy', todo_list, method: :delete, data: { confirm: 'Are you sure?' } %></td> </tr> <% end %> </tbody> </table>
<br>
<%= link_to 'New Todo list', new_todo_list_path %>
new.html.erb
<h1>New todo_list</h1>
<%= render 'form' %>
<%= link_to 'Back', todo_lists_path %>
show.html.erb
<p id="notice"><%= notice %></p>
<p> <strong>Title:</strong> <%= @todo_list.title %> </p>
<p> <strong>Description:</strong> <%= @todo_list.description %> </p>
<%= link_to 'Edit', edit_todo_list_path(@todo_list) %> | <%= link_to 'Back', todo_lists_path %>
create_spec.rb
require 'spec_helper'
describe "Adding todo items" do let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: "Milk"
click_button "Save"
expect(page).to have_content("Added todo list item.")
within("ul.todo_items") do
expect(page).to have_content("Milk")
end
end
end
edit_spec.rb
require 'spec_helper'
describe "Editing todo items" do let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries")} let!(:todo_item) { todo_list.todo_items.create(content: "Milk")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
within("#todo_item_#{todo_item.id}") do
click_link "Edit"
end
fill_in "Content", with: "Lots of Milk"
click_button "Save"
expect(page).to have_content("Saved todo list item.")
todo_item.reload
expect(todo_item.content).to eq("Lots of Milk")
end
it "is unsuccessful with no content" do visit_todo_list(todo_list) within("#todo_item_#{todo_item.id}") do click_link "Edit" end fill_in "Content", with: "" click_button "Save" expect(page).to_not have_content("Saved todo list item.") expect(page).to have_content("Content can't be blank.") todo_item.reload expect(todo_item.content).to eq("Milk") end
it "is unsuccessful with not enough content" do visit_todo_list(todo_list) within("#todo_item_#{todo_item.id}") do click_link "Edit" end fill_in "Content", with: "" click_button "Save" expect(page).to_not have_content("Saved todo list item.") expect(page).to have_content("Content is too short.") todo_item.reload expect(todo_item.content).to eq("Milk") end
end
index_spec.rb
require 'spec_helper'
describe "Viewing todo items" do let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "displays the title of the todo list" do
visit_todo_list(todo_list)
within("h1") do
expect(page).to have_content(todo_list.title)
end
end
it "displays no items when a todo list is empty" do
visit_todo_list(todo_list)
expect(page.all("ul.todo_items li").size).to eq(0)
end
it "displays item content when a todo list has items" do
todo_list.todo_items.create(content: "Milk")
todo_list.todo_items.create(content: "Eggs")
visit_todo_list(todo_list)
expect(page.all("ul.todo_items li").size).to eq(2)
within "ul.todo_items" do
expect(page).to have_content("Milk")
expect(page).to have_content("Eggs")
end
end
end
create_spec.rb (todo_lists)
require 'spec_helper'
describe "Creating todo lists" do
def create_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list"
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New Todo List")
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Create Todo list"
end
it "redirects to todo lists index page on success" do
create_todo_list
expect(page).to have_content("My todo list")
end
it "displays an error when the todo list has no title" do
expect(TodoList.count).to eq(0)
create_todo_list title: ""
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I am doing today")
end
it "displays an error when the todo list has a title with less than 3 characters" do
expect(TodoList.count).to eq(0)
create_todo_list title: "my"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I am doing today")
end
it "displays an error when the todo list has no description" do expect(TodoList.count).to eq(0)
create_todo_list description: ""
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery List")
end
it "displays an error when the todo list has a description with less than 5 characters" do
expect(TodoList.count).to eq(0)
create_todo_list description: "list"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery List")
end
end
destroy_spec.rb
require 'spec_helper'
describe "Deleting todo lists" do let!(:todo_list) {TodoList.create(title: "Groceries", description: "Grocery List")}
it "deletes a todo list entry" do
# todo_list todo_list: todo_list, title: "New Title", description: "New Description"
visit "/todo_lists"
# todo_list.reload
within "#todo_list_#{todo_list.id}" do
click_link "Destroy"
end
expect(page).to_not have_content(todo_list.title)
expect(TodoList.count).to eq(0)
end
end
edit_spec.rb
require 'spec_helper'
describe "Editing todo lists" do let!(:todo_list) {TodoList.create(title: "Groceries", description: "Grocery List")}
def update_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
todo_list = options[:todo_list]
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Update Todo list"
end
it "updates a todo list successfully with correct information" do
update_todo_list todo_list: todo_list, title: "New Title", description: "New Description"
todo_list.reload
expect(page).to have_content("Todo list was successfully updated")
expect(todo_list.title).to eq("New Title")
expect(todo_list.description).to eq("New Description")
end
it "displays an error with too short a description" do
update_todo_list todo_list: todo_list, description: "hi"
expect(page).to have_content("error")
end
end
1 Answer

Richard McGrath
5,597 PointsYou can find what I have the To-do List code here: To-do List Code