Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial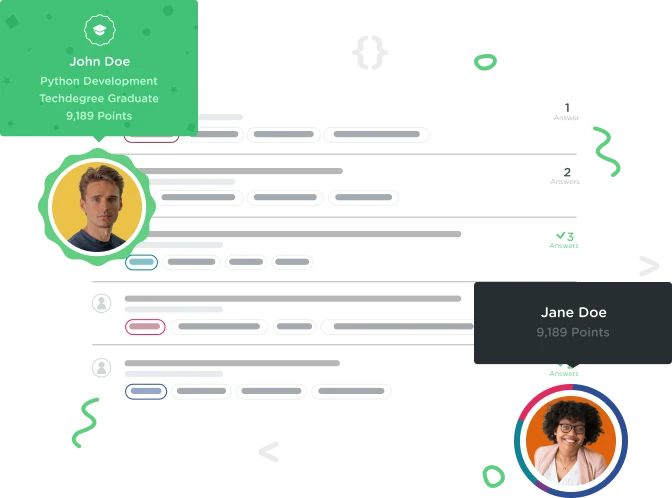

brandonlind2
7,823 PointsCould someone explain switch statements, to me? Why arent they taught in treehouse?
Are they obsolete? If not what benifits do they offer over if else statements and are they necessary to learn?
1 Answer

Casey Ydenberg
15,622 PointsThey aren't quite obsolete, but you do see them fairly rarely. They are very similar to if ... else if ... else but they can be somewhat less verbose and/or easier to read in some circumstances.
Here's an actual example:
function FolderStore() {
this.folders = [];
dispatcher.register(function(payload) {
switch (payload.type) {
case 'GET_FOLDERS':
this.getFolders();
break;
case 'ADD_FOLDER':
this.addFolder(payload.content.name);
break;
default:
break;
}
}.bind(this));
}
In this example, it's very easy to see that the only thing that matters for the various cases is the value of payload.type. If you were using an if ... else ... each expression would be (payload.type === 'something')
. There's nothing wrong with this, of course, but the switch statement puts the important bit right at the top and makes it clear it's the only thing that matters.
The only thing that makes it different from if ... else ... is that tricksy little break
, which you can't ever forget. It's probably possible to do really clever things by leaving it out, but I wouldn't recommend it.
akak
29,445 Pointsakak
29,445 PointsThey are also a good choice when there is a need to map key codes. You may assign more than one case to a certain action. Here's an example from my code:
brandonlind2
7,823 Pointsbrandonlind2
7,823 PointsThanks!
Casey Ydenberg
15,622 PointsCasey Ydenberg
15,622 PointsThat's a much better example.