Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial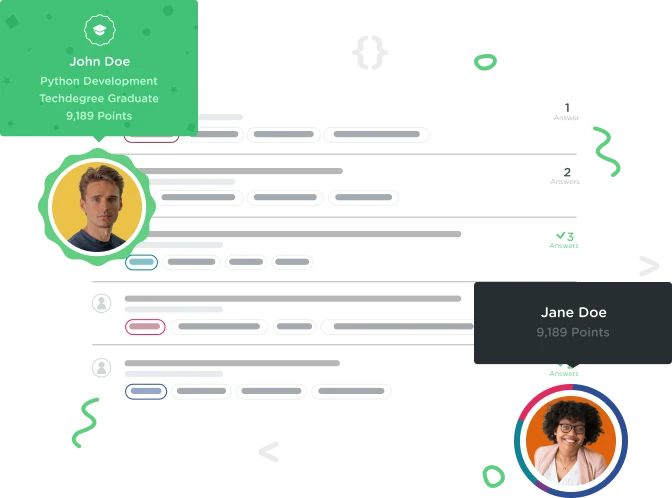
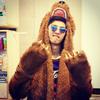
Sonam Wangyal
2,208 PointsCould someone explain what the init method is doing and the syntax of it please?
- (instancetype)initWithIndex: (NSUInteger)index { self = [super init]; if (self){ .... } }
1 Answer
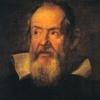
landonferrier
25,097 PointsSonam, throughout your career of being a objective-c programmer you will use alloc and init methods every day. Compared to other computer languages like Java and Ruby, Objective-C gives programmers the best control with memory management and control. The rule of thumb, never call alloc without init or init with alloc, they should always be called together. Modern instantiation of a class will look like this:
MyNewCar *myNewCar = [[MyNewCar alloc] initWithMake:@"BMW" model:@"325i" year:2010];
In that code, you first allocate or create a space in memory for the new instance of the MyNewCar. After the memory has been allocated it is ready for use so you then call init to actually create an instance of the class. Most Apple classes (UIView, UIButton) have many convenience initializers that you can use to reduce code.
These are the exact same, but the first is easier to read and a takes only a fourth of the space in your file,
MyNewCar *myNewCar = [[MyNewCar alloc] initWithMake:@"BMW" model:@"325i" year:2010];
MyNewCar *myNewCar = [[MyNewCar alloc] init];
myNewCar.make = @"BMW";
myNewCar.model = @"325i";
myNewCar.year = @"2010";
If you have any questions or want to know how to make a custom init method, feel free to ask!
Sonam Wangyal
2,208 PointsSonam Wangyal
2,208 PointsThank you, that cleared up a lot for me!
Also for the Build a Playlist app, in the MusicLibrary implementation file, I didn't understand the purpose of the:
I understand that the _library is a instance variable of type NSDictionary, but the part with the self and super I don't quite understand.