Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial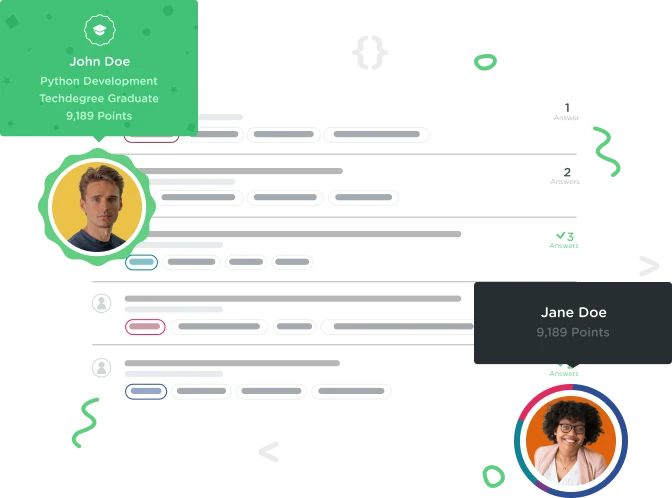
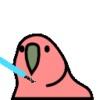
Vincent Nguyen
4,520 PointsCould someone help me with this?
I need to remove vowels from a word but im not sure how to do it
def disemvowel(word):
word = input("What would you like to de-vowel? ")
disemvowel = word.remove('a','A','e','E','i','I','o','O','u','U')
print(disemvowel)
1 Answer

Alex Wootton
Courses Plus Student 5,943 PointsI went with the following solution personally:
- Create a list of
vowels
to match the letters in ourword
against - Convert our
word
string into a list so that we can useremove()
on it - Start a
for
loop that iterates through a copy of theletters
list (this is important, I'll explain at the end) - Check to see if the current
letter
is in thevowels
list in it's lowercase form - If it is, use
remove()
to remove the current letter fromletters
list. Remember that it only removes the first match that it finds - Once each
letter
inletters
has been checked, join each item in theletters
list into a single string -
return
the string. It is important toreturn
and notprint
so that the output can be handed back to it's caller. Some great explanations and examples can be found here
The code will look something like this:
def disemvowel(word):
vowels = ["a","e","i","o","u"] # a list of vowels to compare our letters against
letters = list(word)
for letter in letters.copy():
if letter.lower() in vowels:
letters.remove(letter)
word = "".join(letters)
return word
As promised, the reason we iterate through the letters
list backwards is to ensure that we don't accidentally skip letters by changing their indexes in the list. Here is some code to demonstrate:
A list is indexed from left to right, starting at zero. So if we take the string "aid" for example, once split into a list we have something that looks like this:
["a","i","d"]
With "a"
at index 0
, "i"
at index 1
and "d"
at index 2
If we were to iterate through the list starting at 0
, we would check "a"
against our vowels, match it, remove it and then move on to the next item. However, because we have removed "a"
, "i"
is now the first item in the list and has assumed the index of 0
and following suite "d"
is now at index 1
. The for
loop continues on and checks the item at index 1
so "d"
is checked, not found in the list of vowels and the loop ends as there are no more items in the list to iterate through. As you might have spotted, "i"
was never checked and as such is returned along with "d"
when the function ends.
To prevent this from happening we can simply use a copy of the letters
list that does not change when we remove items from our original.
I hope this helps!
Vincent Nguyen
4,520 PointsVincent Nguyen
4,520 PointsThank you for helping! It helped a lot :)