Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial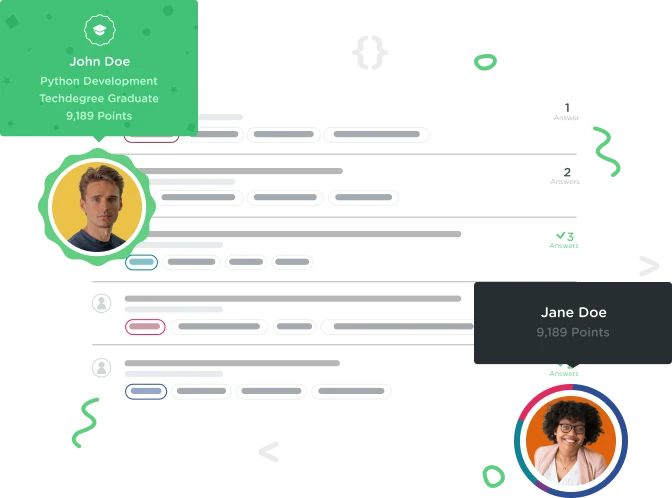

Jay Harper-Harrison
1,044 PointsCould someone help with this basic program?
def us_to_bps(usd):
usd = input("How much would you like to convert?")
amount = usd * 0.60
print("{} US Dollars is equal to {} British pound sterling.".format(usd,amount))
def user_input():
usd = input("How much would you like to convert?")
us_to_bps(usd)
This is a basic currency converter but it does not print anything when I run it. I would appreciate it if someone could help.
1 Answer

Tom Bedford
15,645 PointsHi Jay, you've defined some functions but you haven't called them.
To call the function after you've defined it write the function name:
us_to_bps()
You had a couple of syntax issues too, and you'll need to convert the usd string to an integer in order to multiply it.
Try something like this:
def us_to_bps():
usd = input("How much would you like to convert?")
amount = int(usd) * 0.60
print("{} US Dollars is equal to {} British pound sterling.".format(usd,amount))
us_to_bps()
Jay Harper-Harrison
1,044 PointsJay Harper-Harrison
1,044 PointsThank you.
Jay Harper-Harrison
1,044 PointsJay Harper-Harrison
1,044 PointsDo you know why my code doesn't output anything? If so could you explain?
Tom Bedford
15,645 PointsTom Bedford
15,645 PointsYour original code doesn't output anything as you didn't call either of the functions you created. Have you got it working with the code I suggested?
The key to having it run is the last line
us_to_bps()
which runs the us_to_bps function.Jay Harper-Harrison
1,044 PointsJay Harper-Harrison
1,044 PointsYour code works perfectly. I would like to know why this doesn't work.
It comes out with this error whenever I run it:
python converter.py
Traceback (most recent call last):
File "converter.py", line 6, in <module>
us_to_bps(usd)
NameError: name 'usd' is not defined
Tom Bedford
15,645 PointsTom Bedford
15,645 Pointsus_to_bps(usd)
The brackets are used to pass in arguments for a function, for your function you don't need any arguments as
usd
is set within the function itself.Take out the
usd
arguement from the brackets when you define and callus_to_bps()
and it should run fine.You'll also need to convert the
usd
value that you set in the function to an integer otherwise you'll get another error when you try to multiply using it.Jay Harper-Harrison
1,044 PointsJay Harper-Harrison
1,044 PointsOkay thank you so much for your help.