Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial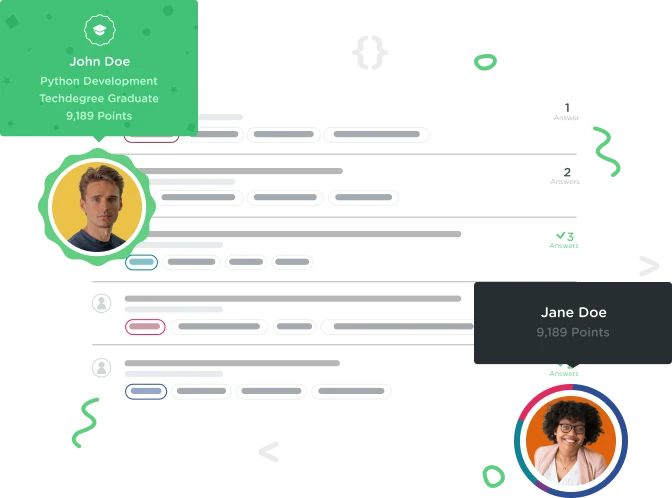

hl9
8,443 PointsCould someone please give an example of "minified" CSS?
Hi,
I'm not quite clear on what it means to minify CSS. Is the following CSS already "minified" or would this much CSS slow down a page? (Here's the HTML first):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>My Page</title>
</head>
<body>
<ol>
<li id="lione"><span id="first">First list item</span></li>
<li id="linext"><span id="next">The next thing</span></li>
<li id="lithird"><span id="third">The third thing</span></li>
<li id="li"><span id="one">This one comes last</span></li>
</ol>
<ul>
<li id="item">First list item</li>
<li id="item next">The next thing
<ul>
<li>First list item</li>
<li>The next thing</li>
<li>The third thing</li>
<li>This one comes last</li>
</ul>
</li>
<li>The third thing</li>
<li>This one comes last</li>
</ul>
</body>
</html>
And here's the CSS I applied:
#lione {
font-size: 2em;
color: steelblue;
}
#first {
font-size: 1em;
color: red;
}
#linext {
font-size: 2em;
color: black;
}
#next {
font-size: 1em;
color: orange;
}
#lithird {
font-size: 2em;
color: blue;
}
#third {
font-size: 1em;
color: pink;
}
#lilast {
font-size: 2em;
color: brown;
}
#one {
font-size: 1em;
color: green;
}
Thank you
7 Answers
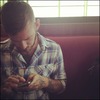
Erik McClintock
45,783 PointsYes, looking through minified code is a nightmare for humans, and that's why we don't do it! Generally, you'll want to have two versions of your code - one that is written for humans, and one that is written for the computer.
In this case, you would have two files: one called style.css, and one called style.min.css.
"style.css" would be this:
#lione {
font-size: 2em;
color: steelblue;
}
#first {
font-size: 1em;
color: red;
}
#linext {
font-size: 2em;
color: black;
}
#next {
font-size: 1em;
color: orange;
}
#lithird {
font-size: 2em;
color: blue;
}
#third {
font-size: 1em;
color: pink;
}
#lilast {
font-size: 2em;
color: brown;
}
#one {
font-size: 1em;
color: green;
}
And would only live on your local machine - you would not need to load this onto the production server AT ALL. Thus, you also do not link to this file from your HTML. Think of this version as the working version of the file. This is where you go to write all original CSS, and make any edits or tweaks along the way.
From your working version ("style.css"), you then save and create a second version that is minified, called "style.min.css". This is the minified, "production" version of your stylesheet, and will be the one that you a) link to in the head section of your HTML, and b) load onto the production server. This is the version that the computer wants, because it is smaller, so your site will load faster, and it doesn't need all the white space and indentation that we humans to to read it.
"style.min.css" would look like this:
#lione{font-size:2em;color:#4682b4}#first{font-size:1em;color:red}#linext{font-size:2em;color:#000}#next{font-size:1em;color:orange}#lithird{font-size:2em;color:#00f}#third{font-size:1em;color:pink}#lilast{font-size:2em;color:brown}#one{font-size:1em;color:green}
Does this make sense? You use the original, working version ("style.css") to write all your CSS and make all edits as necessary, then save it out to your local machine, then you can go to a free CSS minifying service online (such as http://cssminifier.com/), copy and paste in your white-space filled, indented code, and it will spit out a minified version for you to copy and paste into your production version code ("style.min.css"), which is the file that you will link to in the head of your HTML, and the file that you will load to your web server. By doing this, you reduce bloat for your site's load time on the live server, but you retain a nice, easy-to-read version for yourself on your local machine where you can make edits as need be. Just make sure that every time you make edits, you will need to re-minify your CSS, copy/paste it into your style.min.css file, and reload that file. Otherwise, you won't see your edits!
Erik
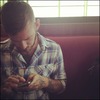
Erik McClintock
45,783 Points"Minifying" your code simply means to strip it of all unnecessary lines and whitespace. For example, the minified version of the CSS you posted above looks like this:
#lione{font-size:2em;color:#4682b4}#first{font-size:1em;color:red}#linext{font-size:2em;color:#000}#next{font-size:1em;color:orange}#lithird{font-size:2em;color:#00f}#third{font-size:1em;color:pink}#lilast{font-size:2em;color:brown}#one{font-size:1em;color:green}
Notice how all unnecessary spaces are removed, and everything runs on one line. This reduces the filesize of the document, and thus will make your pages load faster. For this small amount of CSS, it won't make much of a difference one way or the other, but when you get to having lots of files and larger sites, it can help a lot.
Erik

Ben H
1,114 PointsMost commonly it means removing all whitespace and running it all on one line like so.
lione{font-size: 2em;color: steelblue;}#first{font-size: 1em;color: red;}
Etc...

Ben H
1,114 PointsAlso combining selectors like pi R has mentioned. Essentially removing all whitespace and making it more DRY

hl9
8,443 PointsThank you pi R and Ben for showing me about combining similar values, missed that!

hl9
8,443 PointsHello and thank you everyone for answering so quickly!
I did have a question though -- Erik McClintock -- about putting everything on one line. Wouldn't this make it difficult to read for anyone wanting to update something? The reason I'm asking is I thought Nick or Guil said/showed the importance of making everything sort of neat and tidy and indented, etc. so that things would be easy to read and find.
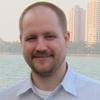
Ryan Field
Courses Plus Student 21,242 PointsWhen you minify CSS (or JavaScript for that matter), it is only for production use. This means that these minified files are created separately and uploaded to your live site, while the easier-to-read larger files are kept locally. When something needs to be changed, you minify the revised file and replace the old style.min.css (or script.min.js) on the live server.

hl9
8,443 PointsOH, this is a brilliant explanation, and solution, thank you Erik!
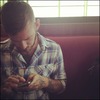
Erik McClintock
45,783 PointsMy pleasure!
Happy coding!
Erik

hl9
8,443 PointsThank you Ryan Field, Wow there is so much to learn! I wonder how many other things I'll find out I need to know but didn't know I needed to know O.o

web kins
8,647 PointsSo you would not recommend going back to the minified file and adding section headings and comments back into the file?