Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial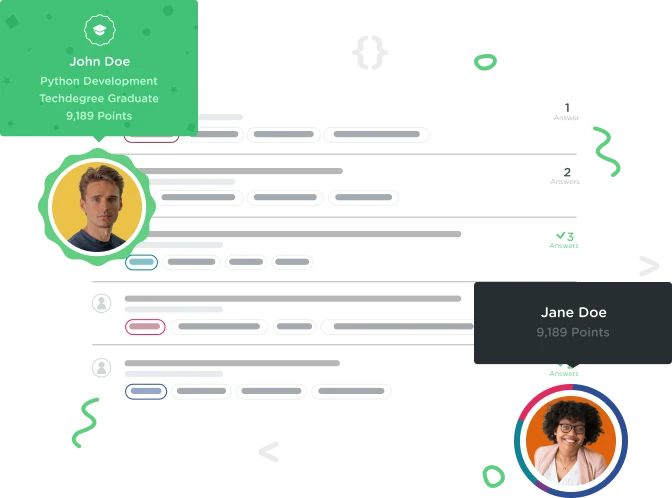

Eli Yazdi
3,639 PointsCould someone please give me a step-by-step explanation of this?
Hi everyone!
I was given this challenge and I have no idea what they mean by the question. Could someone please walk me through this step by step!
The question was "Now set up the prototype chain for the Teacher prototype to inherit from the Person prototype."
Thank you!
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.fullName = function() {
return this.firstName + " " + this.lastName;
};
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.room = roomNumber;
}
3 Answers

LaVaughn Haynes
12,397 PointsYou start off with your constructor function. It's bascially a blueprint that can be used to generate objects. The prototype let's you add properties and methods to the blueprint (and as a result, any objects created from that blueprint). Below I make a blueprint for TheWalkingDead and add a rick method to the prototype.
Then I create a new instance (or copy) of that blueprint called show1. I log it so that you can see the object, and if you click on the arrow in the console you can see the prototype.
//create constructor function...
function TheWalkingDead(){
this.zombies = true;
this.walkers = true;
this.biters = true;
}
//...and add method to prototype
TheWalkingDead.prototype.rick = function(){
console.log('Coral!');
}
//make a new instance
var show1 = new TheWalkingDead();
//log show1 and you can see rick on the prototype
console.log(show1);
//use rick
show1.rick();
Now I'll create a FearTheWalkingDead constructor which will inherit from TheWalkingDead.
//FearTheWalkingDead will inherit properties from
//TheWalkingDead now, but not it's prototype
function FearTheWalkingDead(){
TheWalkingDead.call(this);
}
//make new instance
var show2 = new FearTheWalkingDead();
If you are not familiar with call, the call() method is going to have the effect of taking the code from inside of the TheWalkingDead function and running it inside of the FearTheWalkingDead function like this
function FearTheWalkingDead(){
//TheWalkingDead.call(this);
this.zombies = true;
this.walkers = true;
this.biters = true;
}
//make new instance
var show2 = new FearTheWalkingDead();
As you can see, call did not copy the prototype, just the properties in the constructor function.
//no prototype
console.log(show2);
//trying to use rick would throw an error
//show2.rick();
We need to fix this so that it inherits from the prototype. This is what the test was asking you to do. For the test it wanted you to set it up so that the Teacher prototype inherits from the Person prototype. Here we will set it up so that the FearTheWalkingDead prototype inherits from TheWalkingDead's prototype. For that we will use Object.create(). Object.create creates a new object that inherits from the object passed in as an argument. We want to inherit from TheWalkingDead's prototype so we just need to pass it in as an argument
//make FearTheWalkingDead's prototype inherit
//from TheWalkingDead with Object.create
FearTheWalkingDead.prototype = Object.create(TheWalkingDead.prototype);
//make another instance
var show3 = new FearTheWalkingDead();
//rick is now available!!
console.log(show3);
//use rick
show3.rick();
That's it. Sorry for being long winded. I didn't know which part you didn't understand.
I see someone has answered but I typed all of this up so I'm posting :)
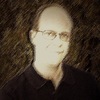
Jason Anders
Treehouse Moderator 145,860 PointsHi Eli,
I answered another student's question on this in this post. I hope this will help you.
Keep Coding! :)

Eli Yazdi
3,639 PointsThank you so much!
Yes, that helps!
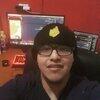
Herman Morales
8,831 PointsTeacher.prototype = Object.create(Person.prototype);
Eli Yazdi
3,639 PointsEli Yazdi
3,639 PointsWow! Thank you so much for taking the time to write all that! This community is really helpful!
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsNo problem. I enjoy it. I found that it's impossible to try to explain something unless you can make it make sense in your own mind. Participating in the forums is helpful not only to people asking questions but also to the person answering the question because it REALLY makes you think about how to explain it (which is harder than one would think). It's a surprisingly good way to learn.