Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial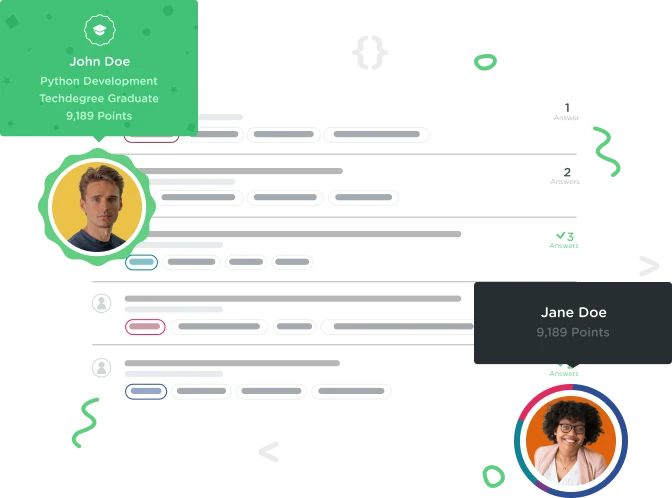

Stephen Poole
9,361 PointsCould someone please remind me what **kwargs actually does?
I don't remember what **kwargs does. Could someone please explain it?
3 Answers

Dan Johnson
40,533 Points**kwargs (short for keyword arguments) is a dictionary who's keys represent argument or attribute names. The two asterisks appended to the front mean that any keyword arguments passed into the function/method will be packed into the dictionary. This way you can pass any number of them as arguments:
class MyClass(object):
my_first_attr = None
my_second_attr = None
def __init__(self, **kwargs):
for key, value in kwargs.items():
if hasattr(self, key):
setattr(self, key, value)
def __str__(self):
return "{} {}".format(
self.my_first_attr,
self.my_second_attr)
example = MyClass(
my_first_attr = "Using packing",
my_second_attr = "to send arguments")
print(example)
You can actually use whatever name you'd like for it, kwargs is just the convention.

Stephen Poole
9,361 PointsOk awesome that makes a lot of sense! Just one more thing though: I thought the double asterisk was only used to unpack dictionaries... So when passing, send="in", whatever="you'd", like="to", to your print_all function, shouldn't you really be doing {"send": "in", "whatever": "you'd", "like": "to"}?
Or is the way you do it just another way of declaring a dictionary?

Dan Johnson
40,533 PointsIt works for both packing and unpacking.
This is kind of a contrived example but it does both:
def pack(**kwargs):
print("{works}".format(**kwargs))
pack(works="for both")

Stephen Poole
9,361 PointsAmazing, that clears everything up. Thanks again Dan, really big help!

Emmet Lowry
10,196 PointsWhen u call items on kwargs what items it refering to and what is the key and value represtent thx

Dan Johnson
40,533 Pointsitems returns a collection of the dictionary's key-value pairs. You can view the key as an index. and the value being whatever is stored at that index:
dictionary = {"Key": "Value"}
# Will print "Value"
print(dictionary["Key"])
Stephen Poole
9,361 PointsStephen Poole
9,361 PointsGreat, Thanks a lot Dan. Do you happen to know where the video is that the instructor did about the packing dictionaries and the use of the double asterisk? I feel like I need to rewatch it because I still find it confusing :/
I tried looking for the video on my own but can't seem to find it anywhere...
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsThe first mention of it I found in this course was in this video. It's mentioned at around 4:40.
Here's a simpler example if the video doesn't help: