Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial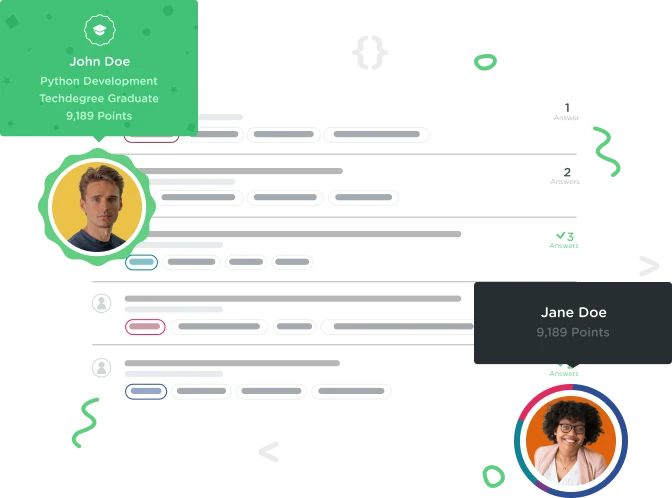
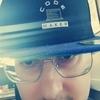
Magnus Martin
44,123 PointsCould you give me a hint where to start?
Please don't give me the answer. I just need an idea where to start.
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo (Object obj) {
if(this.equals(obj)) {
return 0;
}
return 1;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
4 Answers

Michael Barlow
34,317 PointsThere are a couple of things you're missing.
- You need to make sure that you're comparing 2 BlogPost objects. Right now, your method will accept and attempt to compare "this" to any descendant of the Object class (i.e. any object). There's a way to force the parameter to be treated specifically as a BlogPost.
- Check the criteria for ordering 2 posts. You'll need to use the same property on each object to determine what to return in the compareTo() method.
Hope I didn't give too much away! Good luck.

Michael Barlow
34,317 PointsNo problem! ;)
The challenge says to use a BlogPost's creation date as the data for comparison. So in you compareTo() method, you'll need to compare "this" BlogPosts' mCreationDate with that of obj. Since you're comparing Date objects (mCreationDate is an instance of the Date object), they already implement a compareTo() method that will work properly. See the Date Class Documentation and read the details on its compareTo method. So, all you need to do is return that value:
return this.getCreationDate().compareTo(obj.getCreationDate();
This also brings up the answer to #1. If you try to call getCreationDate() on the instance of Object—obj—passed to the function, you'll get an error. That method is only defined for instances of BlogPost. Thus you'll need to cast obj to BlogPost in one of the following ways:
BlogPost objCastToBlogPost = (BlogPost) obj;
OR
return this.getCreationDate().compareTo(((BlogPost) obj).getCreationDate());
Note the formation of the parentheses in the second example. To call BlogPost methods on obj, the syntax should be
((BlogPost) obj).someMethod();
instead of
(BlogPost) obj.someMethod()
The latter will attempt to cast the return of someMethod() to a BlogPost (e.g. getCreationDate()
returns a Date instance, so (BlogPost) obj.getCreationDate()
will try to convert a Date object to a BlogPost object).
I hope this explains it a little better.

Simon Coates
28,694 PointsI think you need to convert the compareTo to use the date and return 0, -1, 1. I think. I'll at least agree that the challenge is not clear.
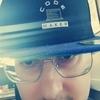
Magnus Martin
44,123 PointsI don't get it. Could you please make me facepalm myself ;)