Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial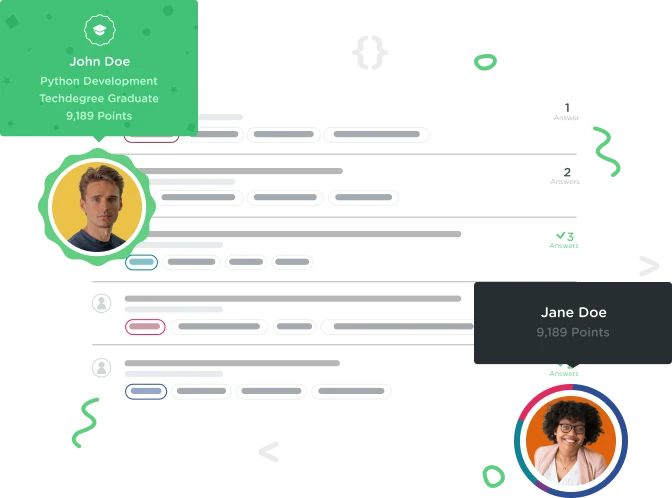

Juan Prieto
976 PointsCould you help me with these I'm a bit confuse
Give me a hand plz
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lastName.charAt(0) == 'a' || 'b' || 'c' || 'd' || 'e' || 'f' || 'g' || 'h' || 'i' || 'j' || 'k' || 'l' || 'm'){
lineNumber +=;
}
else (lastName.charAt(0) == 'n' || 'o' || 'p' || 'q' || 'r' || 's' || 't' || 'u' || 'v' || 'w' || 'x' || 'y' || 'z'){
lineNumber + 1
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
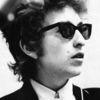
michaelcodes
5,604 PointsHi there! thankfully with characters of letters we can use comparative logic! It would be awfully repetitive if we had to type out an or || for every single condition. We also should normalize the input with a .toLowerCase() method (i am not sure if the challenge calls for this however it is good practice). This would look as follows:
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if(lastName.toLowerCase().charAt(0) < 'n') { //Here we can simply check that its less than n (a-m)
lineNumber = 1; //we also stack the .toLowerCase to normalize input
} else{ //otherwise, return 2
lineNumber = 2;
}
return lineNumber;
}
Also just as a side note, this line of code that you have here:
else (lastName.charAt(0) == 'n' || 'o' || 'p' || 'q' || 'r' || 's' || 't' || 'u' || 'v' || 'w' || 'x' || 'y' || 'z'){
//For this code to work you would need to use "else if"
lineNumber + 1
}
If the condition has logical comparison you want to use "else if" rather than "else". "else" doesn't take any arguments to compare, it is simply the condition that will execute if nothing else matches. Above we used
else{
lineNumber = 2;
}
because in terms of this program, if it isnt a-m it is n-z.
Hope this helps! happy coding
Juan Prieto
976 PointsJuan Prieto
976 PointsWhy are u returning lineNumber after "else" return?
michaelcodes
5,604 Pointsmichaelcodes
5,604 PointslineNumber is the variable we are using to hold the value we want to return. In this case the if checks for (a-m) and sets the lineNumber variable to 1 if so. The else checks for the other cases (n-z) and sets lineNumber to 2 if so. At the end of this else-if we then must return that variable, lineNumber. The method requires this because it is set with an integer return. (public int getLineNumberFor)
Also, the return statement is NOT part of the else. It is after, and will always execute regardless of the outcome from the above if-else