Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial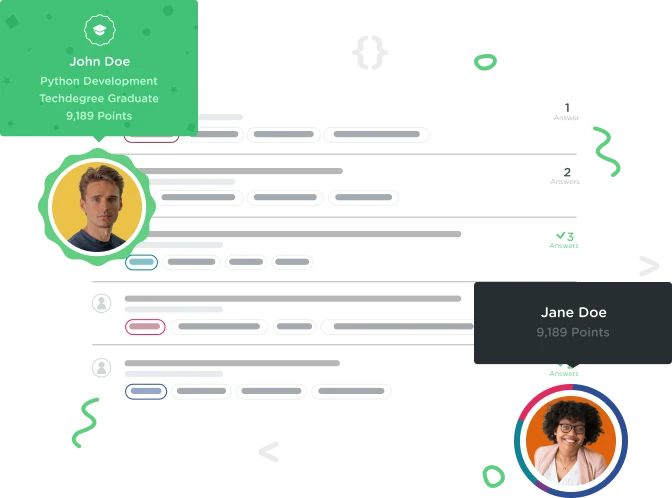

Ed Bundy
5,408 PointsCould you just as easily have used var name instead of const name and made sure that name was local to the function?
I thought variable names are local to functions and therefore you can declare and use the same names within different functions without affecting each other. In that case, it would seem that the error is simply forgetting to use a declaration of a new variable. So the mistake of forgetting to do so would just as easily happen whether you use var or const.
In that case, it seems like a better example of the use of const would be in creating the global variable. Or am I misunderstanding?

Ed Bundy
5,408 PointsActually, I notice in one of the quiz questions a scenario like I was talkng about.
"What happens when this code runs: const taxRate = 8.5; function calculateTax(cost, tax) { taxRate = tax; return (cost * taxRate) / 100; } console.log(calculateTax(100, 10);
"An Uncaught TypeError. is logged to the console" "You can't reassign the value of a constant."
The above code protects taxRate when it is declared outside the function. So there is no concern about using const within the function.
Anyway, I decided to find a workspace and plug in some code.
taxRate = 'mike'; function calculateTax(cost, tax) { var taxRate = 'joe'; return ((cost * tax) / 100); } document.write(calculateTax(100, 10)) document.write( "<br>" + taxRate);
I just changed taxRate to hold strings to put aside the calculations and just zero in on what happens to its value. Whether const or var is used to declare taxRate in the function, the global variable named taxRate is unchanged and prints as mike after the function has been run. So the video seems to be citing the advantage ES2015's const brings to the table by using it within the function, when really it was just his mistake in not forgetting to declare the variable within the function, and whether he used const or var really has no bearing.
I'm new to JavaScript, which is why I'm taking the course. So maybe I'm missing something here, but it seems like a poor example of what const brings to the table with ES2015 (though, I understand const is valuable. I'm surprised that this was something new with EC2015 ).
1 Answer
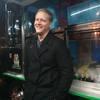
gregory gordon
Full Stack JavaScript Techdegree Student 14,652 Pointsas far as i see you are telling it taxRate = 'mike'; so you will always get mike. But once you set a var inside you are creating a different object.
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsNot really, that's the whole purpose of const - it doesn't implicitly get defined in the global scope, so you can't make the same error with const.
From MDN Const
"Constants are block-scoped, much like variables defined using the let statement. The value of a constant cannot change through reassignment, and it can't be redeclared."