Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial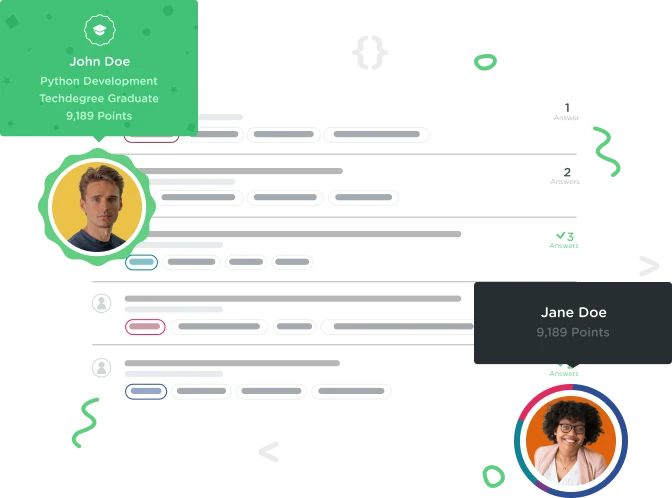
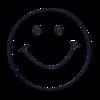
waelitox
2,574 PointsCould you please help identifying the mistake I am making in my code to solve this challenge. I fail to find out.
I keep getting this message in this challenge and I am unable to solve it successfully: Bummer: Hmm, didn't get the expected output. Be sure you're lower casing the string and splitting on all white-space!
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(sentence):
dictionary = {}
sentence.lower()
for word in sentence.split():
dictionary.update({word.lower(): sentence.count(word)})
return dictionary
2 Answers
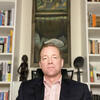
Fergus Clare
12,120 PointsWael Nassief : great question! Are you sure that the interpreter is expecting a .lower() response? Is it possible that by lower casing everything you're causing the interpreter to fail? Lowering cases is helpful, but not always necessary. Consider the following code:
def word_count(sentence):
dictionary = {}
for word in sentence.split():
dictionary.update({word: sentence.count(word)})
return dictionary
#returns this for your sentence string: {'I': 2, 'do': 1, 'not': 1, 'like': 1, 'it': 1, 'Sam': 1, 'Am': 1}
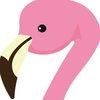
Dave StSomeWhere
19,870 PointsYou mistake is how you are using lower() and split().
# this line doesn't do anything
sentence.lower()
Try testing with below:
print(word_count("I do not like it Sam I Am i I IT it sam SAM"))
# your code returns:
{'i': 4, 'do': 1, 'not': 1, 'like': 1, 'it': 2, 'sam': 1, 'am': 1}
# is should be:
{'i': 4, 'do': 1, 'not': 1, 'like': 1, 'it': 3, 'sam': 3, 'am': 1}
Does that get you rolling - or do you need anything additional
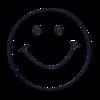
waelitox
2,574 PointsThanks! So your hint helped identify the problem. The solution relies on a piece of information I was missing, which is the return value of the method "count". It returns a List ... not a string. Hence, I did the following and now it works fine!
def word_count(sentence): dictionary = {} sentence = sentence.lower() list_of_sentence = sentence.split() for word in list_of_sentence: dictionary.update({word: list_of_sentence.count(word)}) return dictionary
waelitox
2,574 Pointswaelitox
2,574 PointsDoes not change much, unfortunately.