Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial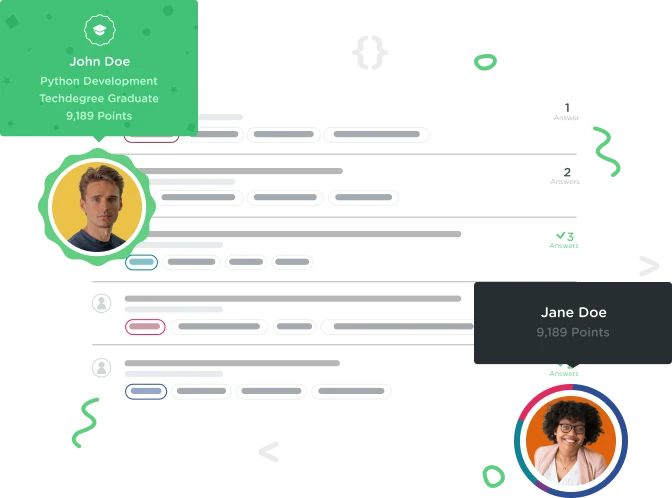

Vedang Patel
7,114 PointsCould you please help me by how to make a user given grid
Could you show me how to make a user input grid I am pretty sure it has something to do with for loops, I just don't know how.
import os
import random
import sys
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4)] # this is where I need the help
def double_space():
print("")
print("")
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations():
return random.sample(CELLS, 3)
def move_player(player, move):
x, y = player
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
return x, y
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
return moves
def draw_map(player):
print(" _"*5)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < 4:
line_end = ""
if cell == player:
output = tile.format("X")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X|")
else:
output = tile.format("_|")
print(output, end=line_end)
def retry():
retry = True
while retry:
retryInput = input("Play again? [Y/n] ").lower()
if retryInput == "y":
game_loop()
elif retryInput == "n":
sys.exit()
else:
clear_screen()
double_space()
print(" ** invalid input **")
double_space()
def game_loop():
monster, door, player = get_locations()
playing = True
while playing:
clear_screen()
draw_map(player)
valid_moves = get_moves(player)
print("You're currently in room {}".format(player))
print("You can move {}".format(", ".join(valid_moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
print("\n ** See you next time! **\n")
break
if move in valid_moves:
player = move_player(player, move)
if player == monster:
double_space()
print(" ** Oh no! The monster got you! Better luck next time! **")
double_space()
playing = False
if player == door:
double_space()
print(" ** You escaped! Congratulations! **")
double_space()
playing = False
else:
if move == "UP" or move == "DOWN" or move == "LEFT" or move == "RIGHT":
double_space()
input(" ** Walls are hard! Don't run into them! **")
double_space()
else:
double_space()
input(" ** Invalid Input **")
double_space()
else:
double_space()
input("press enter to continue ")
retry()
clear_screen()
print("Welcome to the dungeon!")
input("Press return to start!")
clear_screen()
game_loop()
1 Answer
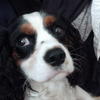
James J. McCombie
Python Web Development Techdegree Graduate 21,199 PointsHello, do you mean something like this?
cells = []
for i in range(5):
for j in range(5):
cells.append((i, j))
this will generate a list of tuples from (0,0), to (4,4), you could of course replace the 5 in the range calls with a variable depending on a users input say.
can also do this with list comprehensions:
cells = [ (i, j) for i in range(5) for j in range(5) ]
James

Vedang Patel
7,114 Pointsthanks
Will Anderson
28,683 PointsWill Anderson
28,683 PointsI really don't understand the question, so I looked over you program. Just from the code it's hard to figure out what the user stories are that you're trying to deliver on. From some of the game's 'screen response coaching' I think it's escaping a monster in a maze. Lots of code there so I ran it in my local IDLE to see what the problem might be. So it runs straight out of the box. Nice! First, you should offer some better instructions to the player. You as the coder know, but let's not keep those gems a secret <wink>. There's a monster in there - running into it you lose :-( , There are walls - the outside perimeter it looks like - and a door which you must find to win. And, while playing, you only know where you are and not where you've been, which might be helpful though you'd probably need to have a tracking array/list and another for loop. Operationally you've done a good job using random to seed the monsters' and the doors' locations. I hope I've addressed at least some of your concerns. Good luck!