Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial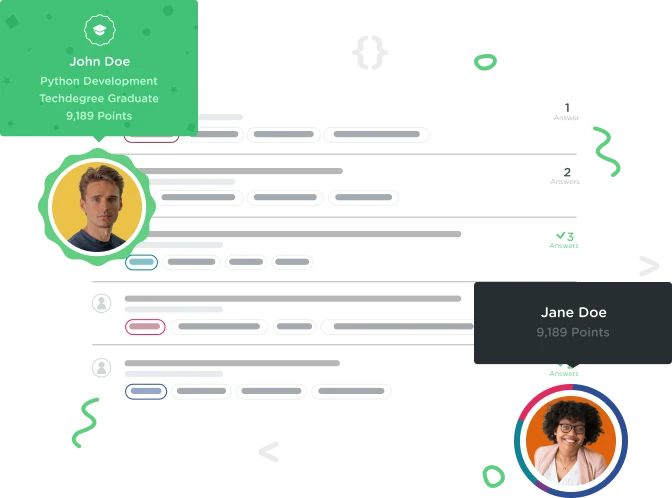

Milagros Roman
1,228 PointsCould you please help me to resolve this error? Bummer! TypeError: 'int' object is not iterable
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
added_list = list()
summarize = 0
def add_list(num):
added_list.append(num)
def summarize(num):
summarize = summarize + add_list[num]
for num in 4:
add_list(num)
summarize(num)
print(summarize)
[edit formating -cf]
6 Answers
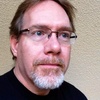
Chris Freeman
Treehouse Moderator 68,423 PointsYour primary error "TypeError: 'int' object is not iterable" is caused by the statement for num in 4
because the for
statement operates on iterable data such as a string, list, tuple, or other object container.
What may not be clear is the structure needed to complete the challenge. When asked to "make a function", the challenge expects a function to be defined to accept certain arguments and return a value. You are not required to print or output the result. The grader program will call the defined function with test values and check the returned value against expected results.
For task 1 of this challenge, you are asked to "Make a function named add_list
that takes a list
. The function should then add all of the items in the list together and return the total."
The would look like:
# define function called "add_list" with one argument
def add_list(input_list):
# initialize result
sum = 0
# Iterate over the input_list to sum elements
for item in input_list:
sum = sum + item
# return results
return sum
For task 2 you are asked "Now, make a function named summarize
that also takes a list. It should return the string "The sum of X is Y.", replacing "X" with the string version of the list and "Y" with the sum total of the list."
# define function called "summarize" with one argument
def summarize(input_list):
# sum elements in input_list using add_list() function
total = add_list(input_list)
# formated string with total: "The sum of X is Y."
string = "The sum of {} is {}.".format(input_list, total)
# return results
return string
As you advance in learning Python programming you will learn to combine statements and use other common idioms.
# For example
# Accumulating to a sum
sum = sum + item
# can be replace by
sum += time
# summarize could be reduced to not-obvious-for-beginners version
def summarize(input_list):
return "The sum of {} is {}.".format(input_list, add_list(input_list))
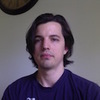
Ryan Merritt
5,789 PointsThe range function is a great way to loop for a specified number of times. For example:
range(5)
returns
[0, 1, 2, 3, 4]
So instead of
for num in 4:
try
for num in range(4):

Milagros Roman
1,228 PointsI tried different things but not successful I would like to have more help because this challenge was not explained and I got confused and I can't advance with other things. thank you

Milagros Roman
1,228 PointsKenneth your course is really very nice but I cannot use the python workplace to exercise because I modify something and I receive error of connection I try again and again and each time error again sorry
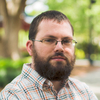
Kenneth Love
Treehouse Guest TeacherYou're getting connection errors all the time?

Chelsea Anna
417 PointsI am having this same issue, although despite this error, I am still receiving some feedback (such as an incorrect output). I'm not sure what's going on.

Milagros Roman
1,228 Pointsthank you. I had finish last Friday in a similar ways as yours. thank you very much Chris Mila
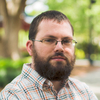
Kenneth Love
Treehouse Guest TeacherYou don't need range()
for this code challenge at all.
John Coolidge
12,614 PointsJohn Coolidge
12,614 PointsIs there a reason during Python challenges I'm asked to use things like total (shown in the above explanation) that I don't ever recall seeing used in the videos thus far? If I've missed it, then that's on me, but it seems I'm asked to use python code that I've not encountered before in order to do the challenges. I'm a complete newbie so how am I to do these challenges when the videos haven't set me up with new terms/code?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsJohn Coolidge, there isn't anything special about
total
in the above example. It is merely a temporary local variable used to hold intermediate results. As shown in the second example, many statements may be combined into a single statement eliminating the use of the local variabletotal
.Breaking up code into multiple statements can help improve readability. In in first example I wanted to explicitly call
add_list()
on a separate line.