Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial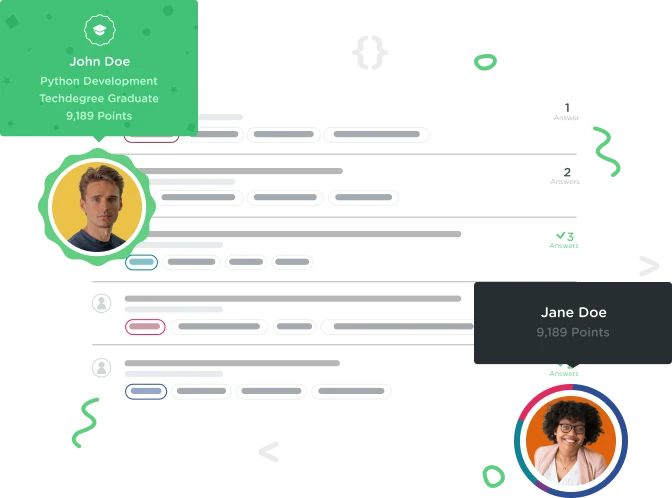

Jake White
41,730 PointsCount the number of belongs_to
I'm working on an internal project management system based off of the todo list course. On the page that lists the todo lists, I want to show the number of items that belongs_to that particular todo list. I've only been using rails for about a week so I'm still trying to figure all of this out. How would I go about doing that?
2 Answers

Kevin Lozandier
Courses Plus Student 53,747 PointsHi, Jake White:
I recommend using size
instead and also consider counter caching instead. This will allow you to speed up the querying of the amount of items of a todo_list considerably (along with using indexes of course).
class TodoList < ActiveRecord::Base
has_many :todo_items
end
class TodoListItems < ActiveRecord::Base
belongs_to :todo_list, counter_cache: true, touch: true
end
Then with rake you would do the following to todo_list :
rails g migration AddTodoItemsCounterCacheToTodoList todo_list_items_count:integer
You then should double check to see if the following occurred inside the migration
add_column :todo_lists, :todo_list_items_count, :integer
If you need to be more specific about what this value is called, you can pass in the the name of what you want the counter cache to be called as a symbol instead of true : counter_cache: :list_count
.
For more information, especially the fact you are a beginner, is to read the official Rails Guide on associations.
In general, I highly recommend reading the complete guide at least once.
With every release, I always go out my way to read all the updated guides at least once affected by the new release and review the other guides at least once a quarter.
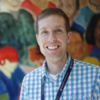
Brandon Barrette
20,485 PointsIf I have a :todo_list, which has_many :todo_list_items (meaning todo_list_item belongs_to :todo_list), you can do:
@todo_list = TodoList.first.todo_list_items.count
#note you'll have to replace the "first" with whatever todo_list you want