Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial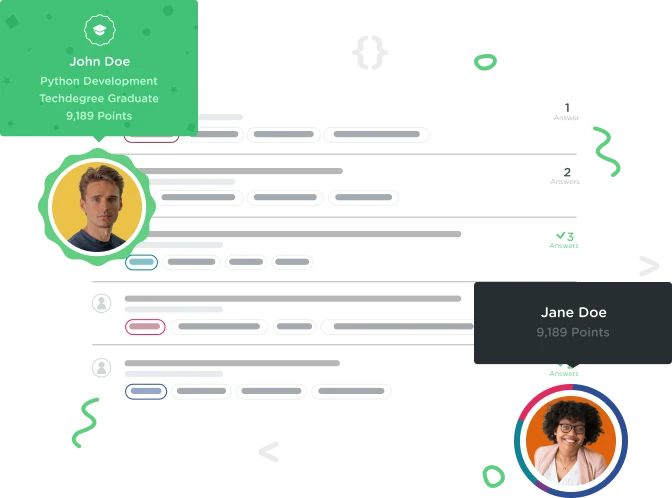

alenwong
4,346 PointsCount the number of occurrence of each word in the sentence
...make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string.
E.g. word_count("I do not like it Sam I Am") gets back a dictionary like: {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1} Lowercase the string to make it easier.
print(word_count("I do not like it Sam I Am")) Result --> {'i': 2, 'do': 1, 'not': 1, 'like': 1, 'it': 1, 'sam': 1, 'am': 1}
As the system didn't provide further information - like the test case it use, the expected result... I can't think of a way to debug this...
Thanks in advance :'(
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(varStr):
varList = varStr.split(" ")
varDict = {}
idx = 0
while idx < len(varList):
varList[idx] = varList[idx].lower()
varDict.update({varList[idx]:0})
idx +=1
for key in varDict.keys():
idx = 0
hit = 0
while idx < len(varList):
if varList[idx] == key:
hit +=1
idx +=1
varDict.update({key:hit})
return varDict
2 Answers
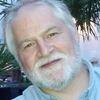
Jeff Muday
Treehouse Moderator 28,717 PointsI said before, it looks like you really understand coding-- which is a great start.
The reason your code snippet did not work is very simple... They are asking to split the sentence on "all whitespace characters" and you are splitting on spaces only, not tabs, linefeeds, etc.
If you remove the double-quoted space character-- you get to the default behavior of the split method, which works on all whitespace (the test cases several types of whitespace characters in between words).
Best of luck with Python!
varList = varStr.split(" ") # change this
varList = varStr.split() # to this
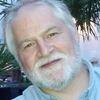
Jeff Muday
Treehouse Moderator 28,717 PointsThat's some nice coding there! It works in my IDE. To me, it looks like you have some background in another language (Java or Javascript). A couple of inefficiencies, but it shows you understand the concept of initialization!
We can simplify it quite a bit approaching it in a more Pythonic way.
def word_count(varStr):
varDict = {}
# iterate over the split string
for word in varStr.split():
# the magic line of code below, the "get" method attempts to get the key,
# if it isn't there, it returns a zero
# learn this method and it will serve you well!
varDict[word.lower()] = varDict.get(word.lower(), 0) + 1
return varDict

alenwong
4,346 PointsHi Jeff
Thanks for the feedback. but specifically I would like to understand why my code can't pass the "word count" test https://teamtreehouse.com/library/python-collections-2/dictionaries/word-count
I'd like to know if there's something wrong with the logic of my code, or something else.
alenwong
4,346 Pointsalenwong
4,346 PointsIt works!! Thanks Jeff :)