Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial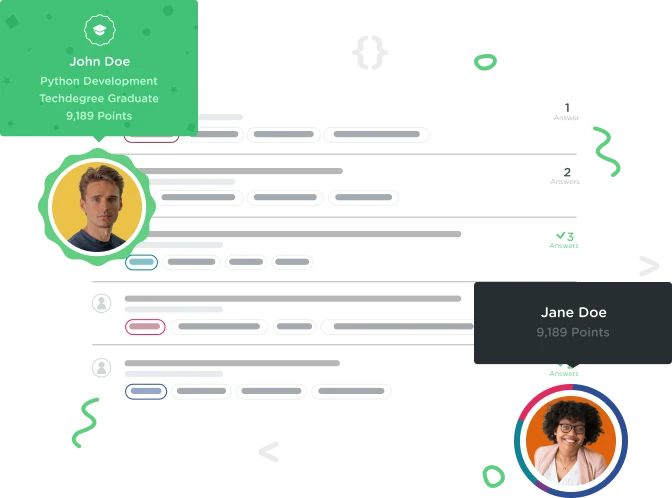

Darrel Lyons
2,991 PointsCountdown using Javascript
How do i create a simple coundown timer using javascript? I set a time in the database as time() + 60. How do i get this to countdown from 60 to zero and then say Done?
1 Answer
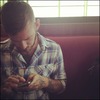
Erik McClintock
45,783 PointsDarrel,
You can achieve this by using a loop (for or while, whichever syntax you prefer) that ticks the timer variable down by X during each iteration, where X is however fast you want the timer to count down. Once your timer variable reaches 0, display your message, again with whatever method you prefer (console.log, alert, etc.).
Erik
Erik McClintock
45,783 PointsErik McClintock
45,783 PointsFor example:
Of course, you could target an element in the document and update its innerHTML with JS at your own discretion, and you'd probably want to wrap the above code in a function and call it a particular time depending on what you need the countdown for, but those are all decisions you would need to make based on the needs of your project.
Erik
Darrel Lyons
2,991 PointsDarrel Lyons
2,991 PointsThanks Eric!! Thanks for using your own time to help me! :)