Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial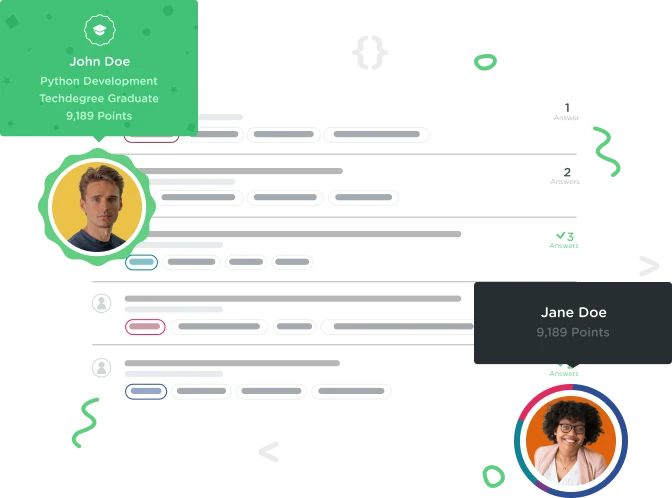
4 Answers

alexlitel
25,059 PointsMantas Gudauskas The parseInt(prompt(question))
method in the for loop is intended to convert the string answer from the prompt into a number. In the video, all the answers are numbers, so the method is appropriate. All your answers, however, are strings, and aside from question two, none of these can be converted into numbers.
So you will need to slightly simplify your code and change the value of the response
variable to the string the user types in the prompt. If you go to the response variable in your for loop and remove parseInt(
method and the second closing parentheses after question
, your code will work. Your loop should look like this:
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
if ( response.toLowerCase() === answer ) {
score += 1;
}
}
If you wanted to use both numbers and strings for answers and use the parseInt method, you could add an OR operator and change your if statement to:
if ( response.toLowerCase() === answer || parseInt(response) === answer )
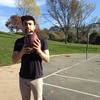
Jason Desiderio
21,811 PointsMantas Gudauskas - the problem looks like it's with your response variable.
Two things:
- Once you run the prompt through parseInt(), it turns into an integer. Since there are no letters, integers don't have a toLowerCase() method - only strings have that method. This is throwing the error which can be seen inside the developer console.
- Not all of your answers in the questions array are integers so it wouldn't be appropriate to use parseInt() on every response.
If you change it to the code below, your program will function as desired
response = prompt(question);

Jeremy Fisk
7,768 PointsI think it should work if you remove the parseInt
from line 20 of you code, and just make it state response = prompt(question);

Mantas Gudauskas
2,610 PointsThanks for the help!!!