Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial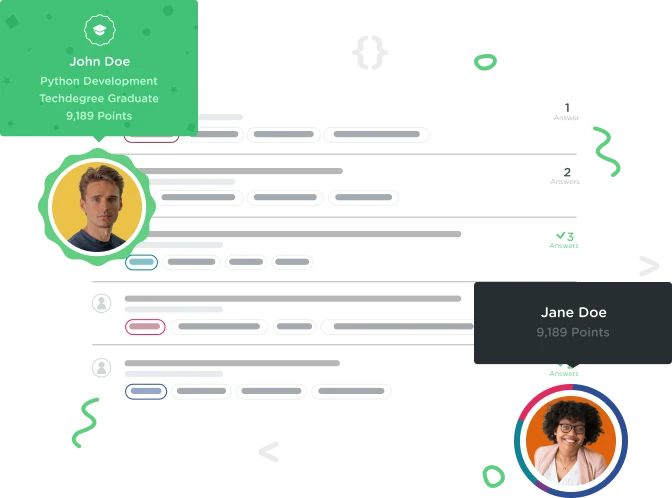

Jeff Miller
651 PointsCounting dictionary keys using a list
I seem to have hit a roadblock on my path to Python stardom. This particular task requests that we "Write a function named members that takes two arguements, a dictionary and a list of keys. Return a count of how many of the keys are in the dictionary". My code so far:
members = [{'name': 'Jeff', 'title': 'GIS'}, ['name', 'age', 'title']]
keys = members.keys()
for keys in members:
return(len(keys))
I think it's the list and dictionary within the list that's throwing me off. Any guidance would be appreciated :)

Jeff Miller
651 PointsThanks Jason. Another rookie mistake on my part...
2 Answers

Duane Moody
18,163 PointsFirstly, be sure to write this as a function.
def members(dictionary, list_of_keys):
The objective is to see if the a key in the list of keys is found in the dictionary. To do this, you will want to iterate over the list (list_of_keys
) and see if any of those keys are found in your dictionary.
Be sure to define a counter variable to add 1 each time you find a key match.
Iterate over list_of_keys
for key in list_of_keys:
Compare each iteration to dictionary
which is your first variable in the function.
if key in dictionary:
Then return your counter
when this is done.
I hope this helps and gets you on the right track.
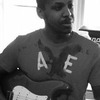
Samuel Webb
25,370 PointsI was so confused until I read this. Thanks.

Jason Anello
Courses Plus Student 94,610 PointsHi Jeff,
I don't see where you have created a members
function. I'll try to give you a high level overview of what the code should be.
You should have something like: def members(my_dict, keys):
to start off with.
You need to have a counter variable which starts off at zero and then gets incremented every time a key is found in the dictionary.
You have a list of keys that are passed in so you want to iterate over that list in a for loop and check if each key from that list is in the dictionary or not. If it is, then you increment your counter variable. The code comment tells you that you can do the key in dict
syntax to check that.
After looping you would then return your counter variable.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Jeff,
I went ahead and fixed your code formatting for you.
See this thread: https://teamtreehouse.com/forum/posting-code-to-the-forum