Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial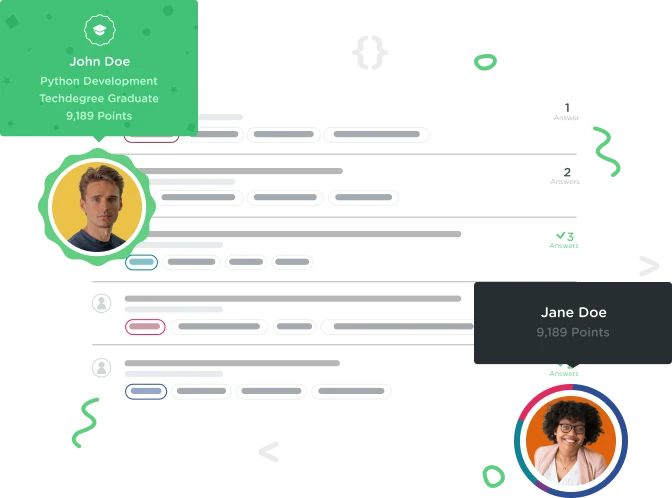

Gensley McD
30 PointsCounting how many lowercase letter a word has
Keep in mind I'm Very Very new at this please Hey guys so thank you in advance for the help. Basically I need to input 3 word I need to store them in a loop Compare which has the Most Lowercase letters and Which has the least Lowercase Letters Using the basics that I have here
So the Input should be like "..... has the maximum number of lowercase: # "..... has the minimum number of lowercase: #
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int lwcase = 0;
int lowcase = 0;
int lowCase1 = 0;
for (int i = 0; i < 3; i++) {
System.out.println("Please input a string:");
String str = input.nextLine();
String st = input.nextLine();
String s = input.nextLine();
for(int k=0; k < str.length(); k++) {
if(Character.isLowerCase(str.charAt(i)))lwcase++;
else if(Character.isLowerCase(st.charAt(i)))lowcase++;
else if(Character.isLowerCase(s.charAt(i)))lowCase1++;
}
if(lwcase > lowcase && lwcase > lowCase1)
System.out.println(+ " has the maximum number of lowercase letters " + );
else if(lowcase > lwcase && lowcase > lowCase1)
System.out.println( + " has the minimum number of lowercase letters " + );
}
}
}
2 Answers

Gensley McD
30 PointsOh ok, that makes more sense. Thank you for the help. I'm sorry if I seem bad at this I appreciate the help.

Simon Coates
28,694 PointsYou should probably split some of this into separate methods. eg. have a method called getLowerCaseInString(String toEvaluate). You're trying to evaluate all of the string at the same time, and given different lengths, the concept is flawed. If they told you to use a loop, it might have been to evaluate each string at a time. You seem to be trying to get three string in a loop that runs three times. Maybe something like:
class Main {
public static void main(String[] args) {
int maxLowerChars = -1;
String maxString = null;
String[] strings = {
"piNeApple", "HORSE", "MISanTHroPe" //could prompt for these
};
for(String toEval: strings){
int lowerChars = getLowerChars(toEval);
if( lowerChars > maxLowerChars) {
maxLowerChars = lowerChars;
maxString = toEval;
}
}
System.out.println("String with most Lower case chars: "+maxString);
}
private static int getLowerChars(String toEval)
{
int count = 0;
for(char c: toEval.toCharArray()) {
if(Character.isLowerCase(c)) {
count++;
}
}
return count;
}
}
(I haven't tested this, so I might have messed things up, but worth taking a look in the event something about it makes sense to you)
Simon Coates
28,694 PointsSimon Coates
28,694 PointsIt's just a guess. Depending on what you're doing, different approaches might make sense. However, the more you're doing in a single method, the more chance it has to be confusing. Some coders have extreme strict rules on method length, or loop nesting depth in a method, or require that the moment you can describe something (eg. count number of lower case letters), it should be in its own method.