Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial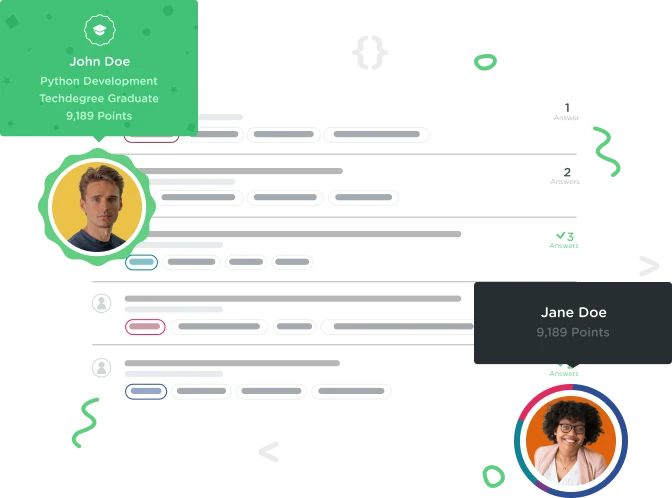

xinzhi zou
661 PointsCounting Scrabble Tiles
for ( char letter: tilesToCharArray())
keeping pop up the symbol error. can not find tiles.
can anyone help me to fix my code appreciate
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char tile){
int count = 0;
for (char letter : tilesToCharArray()) {
if (tile == letter) {
count ++;
}
return count;
}
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
3 Answers
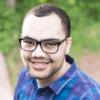
Philip Gales
15,193 PointsTASK 1
Okay, so I need to count how many occurrences of a a specific letter is in the player's tiles. Let's build that over a couple of steps. I've added some example use cases in Example.java
Create a new method named getCountOfLetter that returns an int, and requires a parameter of type char named letter. For this task, just make it return 0.
This tells us we need to create a new method that is 1) named getCountOfLetter 2) returns an int 3) has a parameter named 'letter' that is a 'char' and 4) returns 0.
public int getCountOfLetter (char letter) {
return 0;
}
Your method is correct except for part 3, the parameter. You have to use their names if they tell you what to call something.
TASK 2
You'll need to use your skills to loop through each of the tiles, use an equality check, and then increment a counter if the tile and letter match. You got this!
Now in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method. Make sure to check Example.java for some example uses.
Now we need to 1) 'loop through each of the tiles' 2) use an equality check 3) increment the counter if the tile and letter match and 4) return the number of tiles that match letter (our counter)
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) { //<--------use the name 'letter' here, they told us to
int counter = 0; //<---exactly what you did, good job!
//new variable 'newchar' that loops through the charArray
for (char newtile : tiles.toCharArray()) {
if (newtile == letter) { //<---if the new variable 'newchar' matches the paramter 'letter'
counter ++;
}
}
return counter;
}
}
The things your code was missing are: 1) in your for loop you included the method 'ToCharArray()', but methods need to act on an object. Strings are objects and that is what you correctly chose, but methods should also use a ' . ' and use camelCase (first letter is lower case) so 'tiles.toCharArray()'. 4) you returned the correct variable, but put that return in the for loop. Watch your spacing and it will be easier to find.
tl;dr Correct use of methods 'tiles.toCharArray()' and moving your return statement outside of your for each loop.
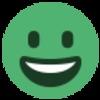
Jack Cummins
17,417 Pointshappy Dee happy times!
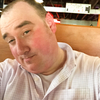
Victor Curtis Jr
5,268 PointsI also needed help on this challenge and this post really helped!! :)
xinzhi zou
661 Pointsxinzhi zou
661 Pointsappreciate Phillip. you answer was very helpful. It also taught me how to think through the answer and get the exactly right answer :)
Ryan Hord
17,688 PointsRyan Hord
17,688 PointsWhy did you choose to use a new variable "newtile" rather than just using "tile"? Is there an advantage or disadvantage to using either one?
Alex Medalla
1,028 PointsAlex Medalla
1,028 PointsI did not get the second task quite well it keeps saying Bummer! Please use an enhanced for loop to cycle through each of the tiles found in tiles.toCharArray could you help me?
Jack Cummins
17,417 PointsJack Cummins
17,417 PointsThank you Philip Gales!