Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial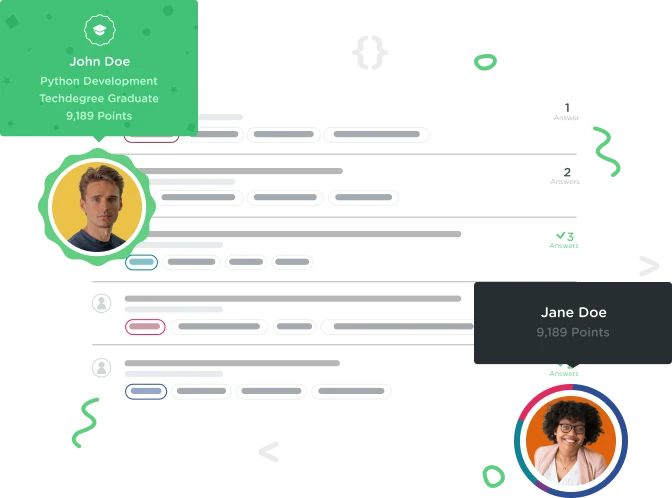

Zachary Vacek
11,133 PointsCounting words in a string using a dictionary - Python Collection Challenge
def word_count(string):
my_string = string.lower().split()
my_dict = {}
for item in my_string:
my_dict[item] = item.count(item)
print(my_dict)
word_count("I am that I am")
The program is running without any errors, but I can't figure out why it isn't properly counting the items in the string.
It should be printing {'i': 2, 'am': 2, 'that': 1}
but it's printing {'i': 1, 'am': 1, 'that': 1}
Can someone help me figure out why this may be?
Thanks!
- Zac
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
3 Answers
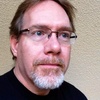
Chris Freeman
Treehouse Moderator 68,423 PointsWhat your original code was doing, as you figured out, was reassigning the dictionary value each time an item
was seen effective only counting the last time it was seen. Also, item.count(item)
will always return 1 on any string.
In your updated solution, you are effectively recounting an item many times, overriding the value with the last time an item
was seen. This parses the full string for each item present. As the string gets longer this becomes much more inefficient. To count the string in a single pass, keep track if the item
is first seen or should increment by 1:
def word_count(string):
my_string = string.lower().split()
my_dict = {}
for item in my_string:
if item in my_dict:
my_dict[item] += 1
else:
my_dict[item] = 1
print(my_dict)

Zachary Vacek
11,133 PointsGot it!
def word_count(string):
my_string = string.lower().split()
my_dict = {}
for item in my_string:
my_dict[item] = my_string.count(item)
print(my_dict)
word_count("I am that I am.")
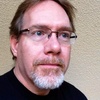
Chris Freeman
Treehouse Moderator 68,423 PointsI promoted this to an answer, but then saw this is a hidden error in the code. See my answer.

Ali Katkar
768 PointswordCounts = {}
for word in string.lower().split():
count = wordCounts.get(word, 0)
wordCounts[word] = count + 1
or simply
words = string.lower().split()
wordCounts = { word: words.count(word) for word in words }