Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial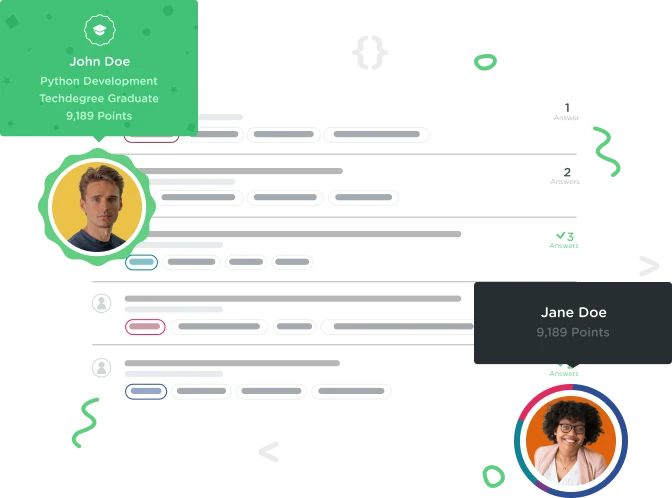
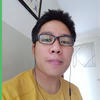
Rifqi Fahmi
23,164 PointsCourse Update ? make fos and oos local variable
We can see in the vide Craig put the fos and oos variable inside the auto-closeable block like this:
try (
FileOutputStream fos = new FileOutputStream("Treet.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
) {
//.......
} catch(IOException ioe) {
//.......
} finally {
// fos and oos variable can't be accessed
}
but if we access the variable inside the "finally" block it will give an error because fos and oos variable are declared in the try block. I have try it on the Intellij IDEA IDE. So I head to google and find the answer https://stackoverflow.com/questions/2854144/problem-with-scopes-of-variables-in-try-catch-blocks-in-java. We should do it like this:
// Local variable scope
FileOutputStream fos;
ObjectOutputStream oos;
try (
fos = new FileOutputStream("Treet.ser");
oos = new ObjectOutputStream(fos);
) {
//.......
} catch(IOException ioe) {
//.......
} finally {
// do something with fos and oos
}
Correct me if i am wrong :) Craig Dennis
UPDATE
I think with this try-with-resource block we don't have to use the finally block because it will be closed regardless of whether the try statement completes normally or abruptly. https://docs.oracle.com/javase/tutorial/essential/exceptions/tryResourceClose.html.
And also the code above is wrong, we can't make local variable and assign it inside the try statement. https://stackoverflow.com/questions/13836486/why-is-declaration-required-in-javas-try-with-resource. So the correct syntax is:
try (
FileOutputStream fos = new FileOutputStream("Treet.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
) {
//.......
} catch(IOException ioe) {
//.......
}