Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial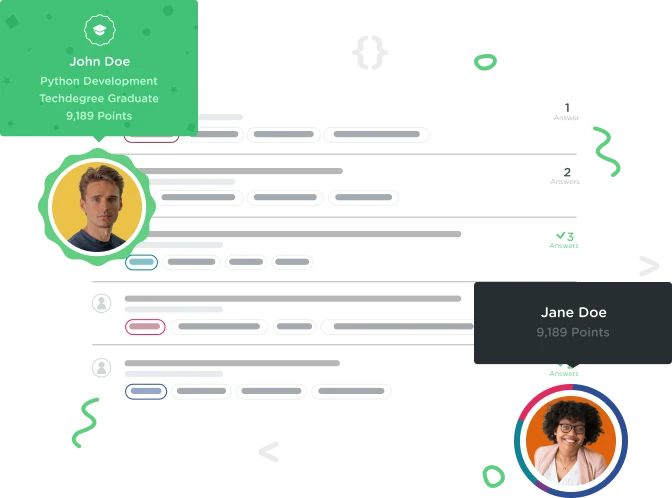

Ibrahim Adeosun
2,973 PointsCrash caused by use of findViewByID method
I am trying to access views from the XML layout in the java activity. I made use of the findViewByID method to do so and be able to access a TextView. Whenever I run the program it c4rashes. The logcat references the line were the findViewByID method is used but I don't seem to get the problem.
package com.example.numberguessinggame;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
TextView a = findViewById(R.id.textView);
Button b = findViewById(R.id.button);
public void changeNum(View view){
a.setText("6 0 0");
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="changeNum"
android:text="Button"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.606" />
</androidx.constraintlayout.widget.ConstraintLayout>
--------- beginning of crash 2019-08-26 18:55:07.304 3605-3605/com.example.numberguessinggame E/AndroidRuntime: FATAL EXCEPTION: main Process: com.example.numberguessinggame, PID: 3605 java.lang.RuntimeException: Unable to instantiate activity ComponentInfo{com.example.numberguessinggame/com.example.numberguessinggame.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'android.view.Window$Callback android.view.Window.getCallback()' on a null object reference at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2718) at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2892) at android.app.ActivityThread.-wrap11(Unknown Source:0) at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1593) at android.os.Handler.dispatchMessage(Handler.java:105) at android.os.Looper.loop(Looper.java:164) at android.app.ActivityThread.main(ActivityThread.java:6541) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767) Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'android.view.Window$Callback android.view.Window.getCallback()' on a null object reference at androidx.appcompat.app.AppCompatDelegateImpl.<init>(AppCompatDelegateImpl.java:249) at androidx.appcompat.app.AppCompatDelegate.create(AppCompatDelegate.java:182) at androidx.appcompat.app.AppCompatActivity.getDelegate(AppCompatActivity.java:520) at androidx.appcompat.app.AppCompatActivity.findViewById(AppCompatActivity.java:191) at com.example.numberguessinggame.MainActivity.<init>(MainActivity.java:18) at java.lang.Class.newInstance(Native Method) at android.app.Instrumentation.newActivity(Instrumentation.java:1173) at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2708) at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2892) at android.app.ActivityThread.-wrap11(Unknown Source:0) at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1593) at android.os.Handler.dispatchMessage(Handler.java:105) at android.os.Looper.loop(Looper.java:164) at android.app.ActivityThread.main(ActivityThread.java:6541) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
2 Answers
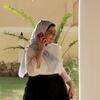
Fatemah Tavakoli
13,797 PointsHi Ibrahim,
your code is fine, however, pay attention to the scope and where to declare and initialize the button and textView. We declare the variables of type TextView and Button outside onCreate and we initialize them inside onCreate.
public class MainActivity extends AppCompatActivity {
TextView a;
Button b;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
a = findViewById(R.id.textView);
b = findViewById(R.id.button);
}
public void changeNum (View view){
a.setText("6 0 0");
}
}

Ibrahim Adeosun
2,973 PointsI tried this and it worked perfectly. Thank you so much!
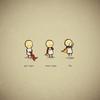
Daniel Prowse
7,941 PointsTry adding a cast before hand maybe?

Ibrahim Adeosun
2,973 PointsI have also tried that and had no luck
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherIn the
xml
, is the name of the TextView and layout the same as being referenced in the above code? Is the activity name the same?