Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial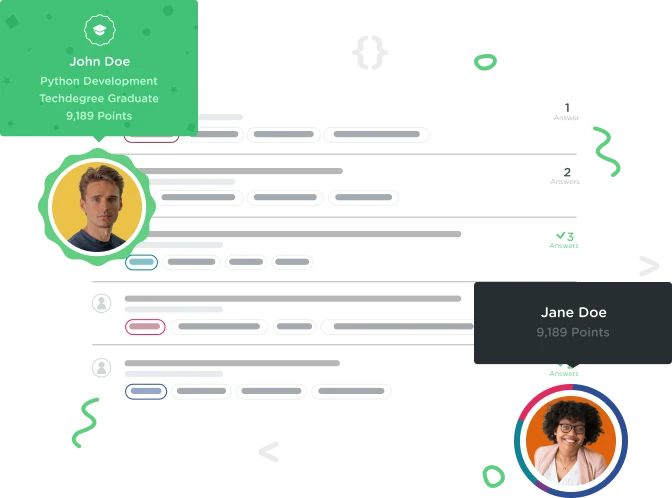

Christopher Pennington
Courses Plus Student 1,384 PointsCrashing after touching Start Button (was working fine)
I've done something, and I've tried for about 3 days to figure it out. I am getting the following error message in logcat:
.RuntimeException: Unable to start activity ComponentInfo(com.example.chris.spaceadventure/com.example.chris.adventurestory.UI.StoryActivity): java.lang.NullPointerException:Attempt to invoke virtual method 'void android.widget.Button.setText(java.lang.CharSequence)' on a null object reference
When I click the START YOUR ADVENTURE button after entering my name, I get the message: "Unfortunately, Adventure Story has stopped"(I have had to start over a couple of times, so I have a different name for this app).
Just let me know what you need to see code-wise to help me figure this one out.
13 Answers
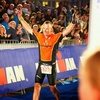
Steve Hunter
57,712 PointsYES!!!
You've not changed your button name - so you haven't initialised mChoice2
:
mChoice1 = (Button)findViewById(R.id.choiceButton1);
mChoice1 = (Button)findViewById(R.id.choiceButton2); //<<-- change to mChoice2 =
Steve.
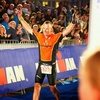
Steve Hunter
57,712 PointsHi Christopher,
I think the error is in StoryActivity
not MainActivity
. Can you post that code too, please? (Java and the XML)
You're pressing the button from MainActivity
which is then calling startStory()
method which invokes the new Activity; that's what's failing. There's something in there that's pointing at a null
; probably a Button and probably when a setText
method is called on it.
Thanks,
Steve.
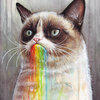
Martin Joubert
4,379 PointsHi Christopher,
Without seeing your actual code it's a bit difficult to help you. When asking a question try to include some of your code to make it easier for others to help :)
By looking at the following:
.RuntimeException: Unable to start activity ComponentInfo(com.example.chris.spaceadventure/com.example.chris.adventurestory.UI.StoryActivity): java.lang.NullPointerException:Attempt to invoke virtual method 'void android.widget.Button.setText(java.lang.CharSequence)' on a null object reference
I'm guessing that you're not initialising your button and thus getting a NullPointerException
.
Make sure that you have initialised your button before trying to set the text.
yourButton = (Button) findViewById(R.id.yourButton);

Christopher Pennington
Courses Plus Student 1,384 PointsMain Activity:
public class MainActivity extends AppCompatActivity {
private EditText mNameField;
private Button mStartButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mNameField = (EditText)findViewById(R.id.nameEditText);
mStartButton = (Button) findViewById(R.id.startButton);
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = mNameField.getText().toString();
startStory(name);
}
});
}
private void startStory(String name){
Intent intent = new Intent(this, StoryActivity.class);
intent.putExtra("name", name);
startActivity(intent);
}
}
[MOD: edited code blocks]

Christopher Pennington
Courses Plus Student 1,384 PointsThe other reference to the StartButton is in
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".UI.MainActivity"
android:background="@android:color/white">
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/titleImageView"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/main_title"
android:scaleType="fitXY"
android:adjustViewBounds="true"
android:contentDescription="SignalsfromMars"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="START YOUR ADVENTURE"
android:id="@+id/startButton"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="30sp"
android:background="@android:color/white"
android:textColor="#3a8aec"
/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:ems="10"
android:id="@+id/nameEditText"
android:layout_above="@+id/startButton"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24sp"
android:hint="Enter Your Name Here to Begin"
android:maxLength="30"
android:background="@android:color/white"
android:textColor="#3a8aec"/>
</RelativeLayout>
Could this be a getters/setters thing?

Christopher Pennington
Courses Plus Student 1,384 PointsThanks for all the help, folks.. here's the StoryActivity:
public class StoryActivity extends Activity {
public static final String TAG = StoryActivity.class.getSimpleName();
public String name;
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private String mName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
mName = intent.getStringExtra("name");
if (mName == null) {
mName = "Friend";
}
Log.d(TAG, mName);
mImageView = (ImageView)findViewById(R.id.storyImageView);
mTextView = (TextView)findViewById(R.id.storyTextView);
mChoice1 = (Button)findViewById(R.id.choiceButton1);
mChoice1 = (Button)findViewById(R.id.choiceButton2);
loadPage();
}
private void loadPage (){
Page page = mStory.getPage(0);
//Drawable drawable = getResources().getDrawable(page.getmImageId());
//Drawable drawable = ContextCompat.getDrawable(this, page.getmImageId());
Drawable drawable = ResourcesCompat.getDrawable(getResources(), page.getImageId(), null);
mImageView.setImageDrawable(drawable);
String pageText = page.getText();
//Add the name if placeholder included. Won't add if no placeholder
pageText = String.format(pageText, mName);
mTextView.setText(page.getText());
mChoice1.setText(page.getChoice1().getText());
mChoice2.setText(page.getChoice2().getText());
}
}
[MOD: edited code block. sh]
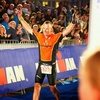
Steve Hunter
57,712 PointsCool - can you give us the XML for that activity too, please?

Christopher Pennington
Courses Plus Student 1,384 PointsIs this what you're after? VVV
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="fill_parent"
android:layout_height="match_parent"
tools:context=".UI.StoryActivity"
android:paddingLeft="0dp"
android:paddingRight="0dp"
android:paddingBottom="0dp"
android:paddingTop="0dp"
android:nestedScrollingEnabled="false"
android:background="@android:color/white">
<ImageView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/storyImageView"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/page0"
android:nestedScrollingEnabled="true"
android:scaleType="fitXY"
android:adjustViewBounds="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low.""
android:id="@+id/storyTextView"
android:layout_below="@+id/storyImageView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24dp"
android:paddingLeft="30dp"
android:paddingRight="30dp"
android:paddingTop="15dp"
android:lineSpacingMultiplier="1.2"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="STOP TO INVESTIGATE"
android:id="@+id/choiceButton2"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:background="@android:color/white"
android:textColor="#3a8aec"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="CONTINUE HOME TO EARTH"
android:id="@+id/choiceButton1"
android:layout_above="@+id/choiceButton2"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:background="@android:color/white"
android:textColor="#3a8aec"/>
</RelativeLayout>
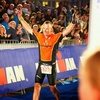
Steve Hunter
57,712 PointsYep - that's what I wanted but it doesn't help.
Can you paste more of the error - there may be a line number in there somewhere that might give us a clue. I can't see that there's anything wrong with the Buttons, which the error implies there is, so it may be somewhere else.
Thanks,
Steve.

Christopher Pennington
Courses Plus Student 1,384 PointsWhat about the null item in getDrawable()?
I went in search of an alternative to the depricated getDrawable() and found:
Drawable: getDrawable(int id) This method was deprecated in API level 22. Use getDrawable(int, Theme) instead.
It's looking for an int, but I'm passing in page.getImageId(). I have no idea what it wants when it asks for a "Theme".

Christopher Pennington
Courses Plus Student 1,384 Points05-24 06:41:47.238 2763-2763/com.example.chris.adventurestory D/AndroidRuntime: Shutting down VM
05-24 06:41:47.238 2763-2763/com.example.chris.adventurestory E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.chris.adventurestory, PID: 2763
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.chris.adventurestory/com.example.chris.adventurestory.UI.StoryActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setText(java.lang.CharSequence)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2325)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2387)
at android.app.ActivityThread.access$800(ActivityThread.java:151)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1303)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5254)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:903)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:698)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setText(java.lang.CharSequence)' on a null object reference
at com.example.chris.adventurestory.UI.StoryActivity.loadPage(StoryActivity.java:67)
at com.example.chris.adventurestory.UI.StoryActivity.onCreate(StoryActivity.java:48)
at android.app.Activity.performCreate(Activity.java:5990)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1106)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2278)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2387)
at android.app.ActivityThread.access$800(ActivityThread.java:151)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1303)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5254)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:903)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:698)
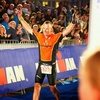
Steve Hunter
57,712 PointsCan you turn on line numbers in Android Studio and let me know which lines are StoryActivity.java:67
and StoryActivity.java:48
, please? Those lines are throwing errors ...
The added null
in the getDrawable
may be something to do with this - I'm not really sure!! We'll get to the bottom of it, though!

Christopher Pennington
Courses Plus Student 1,384 PointsYou're apparently right about the error being in StoryActivity.java.
line 67 is:
mChoice2.setText(page.getChoice2().getText());
line 48 is:
loadPage();

Christopher Pennington
Courses Plus Student 1,384 PointsHA! Thanks for sticking with it, Steve! Yeah... well, I should have seen it in the 3 days I was trying to find my goof. Awesome! I guess the upside is that I re-watched most of the videos in this tutorial.
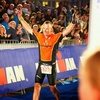
Steve Hunter
57,712 Points
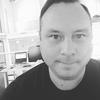
jeremiahjacquet
16,885 PointsI'm having the same problem where I press the "START YOUR ADVENTURE" button and the app crashes. It appears to be several things for me:
- Error inflating class Button
- File res/color/abc_secondary_text_material_dark.xml from drawable resource ID #0x7f0b004f
- <item> tag requires a 'drawable' attribute or child tag defining a drawable
All of the comment above showing a large addition of code to the StoryActivity class, what video-tutorial was that in?
Here is my stack trace:
<p>
Process: com.example.thejacquet3.interactivestory, PID: 2423
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.thejacquet3.interactivestory/com.example.thejacquet3.interactivestory.ui.StoryActivity}: android.view.InflateException: Binary XML file line #18: Binary XML file line #37: Error inflating class Button
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2416)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.view.InflateException: Binary XML file line #18: Binary XML file line #37: Error inflating class Button
at android.view.LayoutInflater.inflate(LayoutInflater.java:539)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at android.support.v7.app.AppCompatDelegateImplV7.setContentView(AppCompatDelegateImplV7.java:280)
at android.support.v7.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.thejacquet3.interactivestory.ui.StoryActivity.onCreate(StoryActivity.java:18)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.view.InflateException: Binary XML file line #37: Error inflating class Button
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:782)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:704)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:835)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.parseInclude(LayoutInflater.java:971)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:831)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.inflate(LayoutInflater.java:515)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at android.support.v7.app.AppCompatDelegateImplV7.setContentView(AppCompatDelegateImplV7.java:280)
at android.support.v7.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.thejacquet3.interactivestory.ui.StoryActivity.onCreate(StoryActivity.java:18)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.content.res.Resources$NotFoundException: File res/color/abc_secondary_text_material_dark.xml from drawable resource ID #0x7f0b004f
at android.content.res.Resources.loadDrawableForCookie(Resources.java:2640)
at android.content.res.Resources.loadDrawable(Resources.java:2540)
at android.content.res.TypedArray.getDrawable(TypedArray.java:870)
at android.view.View.<init>(View.java:3948)
at android.widget.TextView.<init>(TextView.java:677)
at android.widget.Button.<init>(Button.java:109)
at android.widget.Button.<init>(Button.java:105)
at android.support.v7.widget.AppCompatButton.<init>(AppCompatButton.java:62)
at android.support.v7.widget.AppCompatButton.<init>(AppCompatButton.java:58)
at android.support.v7.app.AppCompatViewInflater.createView(AppCompatViewInflater.java:109)
at android.support.v7.app.AppCompatDelegateImplV7.createView(AppCompatDelegateImplV7.java:980)
at android.support.v7.app.AppCompatDelegateImplV7.onCreateView(AppCompatDelegateImplV7.java:1039)
at android.support.v4.view.LayoutInflaterCompatHC$FactoryWrapperHC.onCreateView(LayoutInflaterCompatHC.java:44)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:746)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:704)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:835)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.parseInclude(LayoutInflater.java:971)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:831)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.inflate(LayoutInflater.java:515)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at android.support.v7.app.AppCompatDelegateImplV7.setContentView(AppCompatDelegateImplV7.java:280)
at android.support.v7.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.thejacquet3.interactivestory.ui.StoryActivity.onCreate(StoryActivity.java:18)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: org.xmlpull.v1.XmlPullParserException: Binary XML file line #18: <item> tag requires a 'drawable' attribute or child tag defining a drawable
at android.graphics.drawable.StateListDrawable.inflateChildElements(StateListDrawable.java:182)
at android.graphics.drawable.StateListDrawable.inflate(StateListDrawable.java:115)
06-01 10:33:29.392 2423-2433/com.example.thejacquet3.interactivestory I/art: Background partial concurrent mark sweep GC freed 689(178KB) AllocSpace objects, 0(0B) LOS objects, 23% free, 12MB/16MB, paused 9.537ms total 21.769ms
</p>

Christopher Pennington
Courses Plus Student 1,384 PointsHey, Jeremiah, can you post some of the code you used for the Drawable? My code was from the Mars Interactive Adventure app tutorial, and my issue turned out to be a single character typo concerning a button. Sometimes, itty bitty errors can cause really big error logs. :)
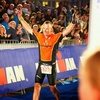
Steve Hunter
57,712 PointsHI there,
The issue seems to be originating, or involving,one particular line of code in the onCreate
method of StoryActivity
: onCreate(StoryActivity.java:18)
BUT, there's some mention of XML issues and the error could be in other areas, as we saw with Christopher's problem that's the subject of this thread.
So ... let's start with posting StoryActivity.java
and highlighting line 18. If that involves a new Intent moving to a new activity, post that new activity too! And if you can post the XML of both activities, that would be great too.
We should be able to have a better idea of the challenge with that lot!!
Steve.
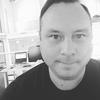
jeremiahjacquet
16,885 Pointspackage com.example.thejacquet3.interactivestory.ui;
import android.content.Intent;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.support.v4.content.res.ResourcesCompat;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.thejacquet3.interactivestory.R;
import com.example.thejacquet3.interactivestory.model.Page;
import com.example.thejacquet3.interactivestory.model.Story;
public class StoryActivity extends AppCompatActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
public String name;
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private String mName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
String name = intent.getStringExtra(getString(R.string.key_name));
if (name == null) {
name = "Friend";
}
Log.d(TAG, name);
}
}
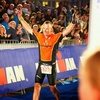
Steve Hunter
57,712 PointsCan you post MainActivity too, please? I'm not sure why the error points to the onCreate
method of StoryActivity
at line 18 when line 18 isn't anywhere near that method?! But it is something to do with the intent between the activities, I think. So let's check the 'outbound' one in Main as well.
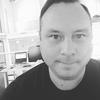
jeremiahjacquet
16,885 PointsHi sorry for the late response.
Here is my MainActivity.java
<p>
package com.example.thejacquet3.interactivestory.ui;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import com.example.thejacquet3.interactivestory.R;
public class MainActivity extends AppCompatActivity {
private EditText mNameField;
private Button mStartButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mNameField = (EditText) findViewById(R.id.nameEditText);
mStartButton = (Button) findViewById(R.id.startButton);
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = mNameField.getText().toString();
startStory(name);
}
});
}
private void startStory(String name){
Intent intent = new Intent(this, StoryActivity.class);
intent.putExtra(getString(R.string.key_name), name);
startActivity(intent);
}
}
</p>
and here is the xml for the activity_main.xml
<p>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:showIn="@layout/activity_main"
tools:context=".ui.MainActivity">
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/titleImageView"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@mipmap/main_title"
android:scaleType="fitXY"
android:adjustViewBounds="true"
android:contentDescription="Signals from Mars"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="START YOUR ADVENTURE"
android:id="@+id/startButton"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:background="#ffffff"
android:textColor="#FF3A8EAE"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:ems="10"
android:id="@+id/nameEditText"
android:layout_above="@+id/startButton"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:hint="Enter your name to begin"
android:maxLength="30"/>
</RelativeLayout>
</p>
and here is the activity_story.xml
<p>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context=".ui.StoryActivity"
tools:showIn="@layout/activity_story"
android:background="#ffffff">
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/storyImageView"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@mipmap/page0"
android:scaleType="fitXY"
android:adjustViewBounds="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low"
android:id="@+id/storyTextView"
android:layout_below="@+id/storyImageView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:paddingLeft="30dp"
android:paddingRight="30dp"
android:paddingTop="15dp"
android:lineSpacingMultiplier="1.2"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Stop and Investigate"
android:id="@+id/choiceButton2"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:background="@color/abc_secondary_text_material_dark"
android:textColor="#3a8eae"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Continue home to Earth"
android:id="@+id/choiceButton1"
android:layout_above="@+id/choiceButton2"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:background="@color/abc_background_cache_hint_selector_material_light"
android:textColor="#3a8eae"/>
</RelativeLayout>
</p>
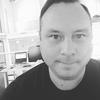
jeremiahjacquet
16,885 PointsOK, When I comment out the the 2 buttons in the activity_story.xml
the app doesn't crash goes. Instead it uses the TextView android:id="@+id/storyTextView"
, but of course without the buttons showing.
Went back and followed the video instructions again and this time the only thing that changed was using the system white for background. I also ensured that all the buttons were using the same hexadecimal color code for all the buttons and viola, it worked!
that seems very touchy to me that using android:background="@color/abc_background_cache_hint_selector_material_light"
would cause a crash instead of android:background="@android:color/white"
In either case its working for now.
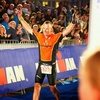
Steve Hunter
57,712 PointsThat seems very strange, I agree!
Glad you got going again ...
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI should have seen that as soon as you posted the
StoryActivity
code - apologies. But the line numbers lead me to the issue. That should work for you now.