Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial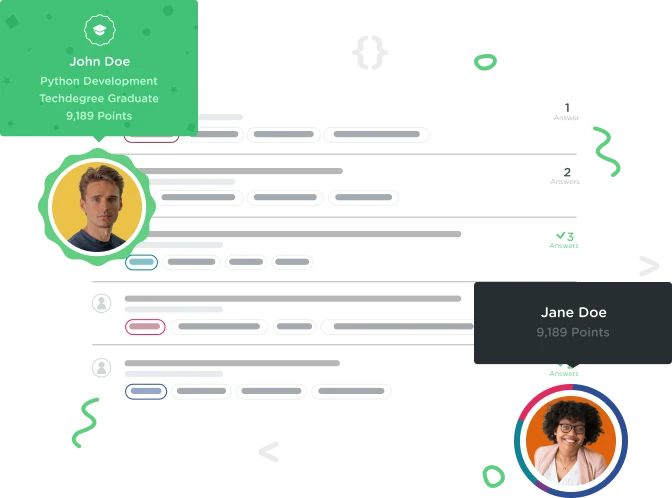

Mark Weiser
2,093 PointsCrashing and getting an error off of the code from video
fatal error: unexpectedly found nil while unwrapping an Optional value (lldb)
because of this line:
let cell = tableView.dequeueReusableCellWithIdentifier("WeatherCell") as! UITableViewCell
within this code:
import UIKit
class WeeklyTableViewController: UITableViewController {
@IBOutlet weak var currentTemperatureLabel: UILabel?
@IBOutlet weak var currentWeatherIcon: UIImageView?
@IBOutlet weak var currentPrecipitationLabel: UILabel?
@IBOutlet weak var currentTemperatureRangeLabel: UILabel?
// Location coordinates
let coordinate: (lat: Double, lon: Double) = (37.8267,-122.423)
// TODO: Enter your API key here
private let forecastAPIKey = ""
var weeklyWeather: [DailyWeather] = []
override func viewDidLoad() {
super.viewDidLoad()
configureView()
retrieveWeatherForecast()
}
func configureView() {
// Set table view's background view property
tableView.backgroundView = BackgroundView()
// Change the font and size of nav bar text
if let navBarFont = UIFont(name: "HelveticaNeue-Thin", size: 20.0) {
let navBarAttributesDictionary: [NSObject: AnyObject]? = [
NSForegroundColorAttributeName: UIColor.whiteColor(),
NSFontAttributeName: navBarFont
]
navigationController?.navigationBar.titleTextAttributes = navBarAttributesDictionary
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
// Return the number of sections.
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// Return the number of rows in the section.
return weeklyWeather.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("WeatherCell") as! UITableViewCell
let dailyWeather = weeklyWeather[indexPath.row]
cell.textLabel?.text = dailyWeather.day
return cell
}
// MARK: - Weather Fetching
func retrieveWeatherForecast() {
let forecastService = ForecastService(APIKey: forecastAPIKey)
forecastService.getForecast(coordinate.lat, lon: coordinate.lon) {
(let forecast) in
if let weatherForecast = forecast,
let currentWeather = weatherForecast.currentWeather {
dispatch_async(dispatch_get_main_queue()) {
if let temperature = currentWeather.temperature {
self.currentTemperatureLabel?.text = "\(temperature)º"
}
if let precipitation = currentWeather.precipProbability {
self.currentPrecipitationLabel?.text = "Rain: \(precipitation)%"
}
if let icon = currentWeather.icon {
self.currentWeatherIcon?.image = icon
}
self.weeklyWeather = weatherForecast.weekly
if let highTemp = self.weeklyWeather.first?.maxTemperature,
let lowTemp = self.weeklyWeather.first?.minTemperature {
self.currentTemperatureRangeLabel?.text = "↑\(highTemp)º↓\(lowTemp)º"
}
self.tableView.reloadData()
}
}
}
}
}
Brian Steele
23,060 PointsBrian Steele
23,060 PointsYou need to change the ! in your as statement to an ?. This should fix the problem or at least prevent crashes.