Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial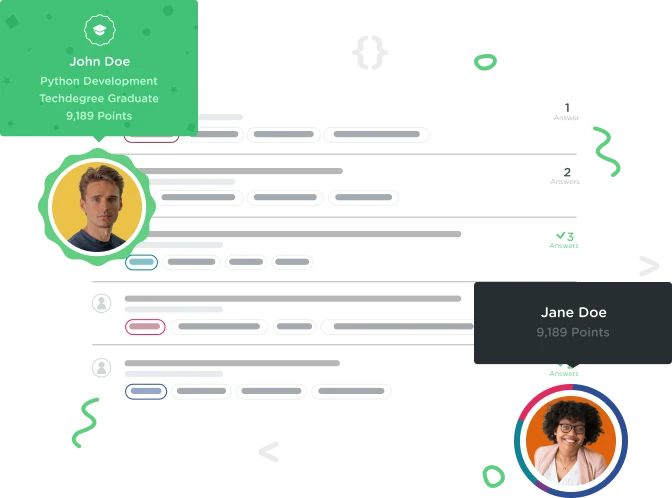

Samuel Igborgbor
4,335 PointsCreate a 'do...while' loop
This is the code we used in the last code challenge. After learning about do...while loops, don't you think this would work better in the do...while style? Re-write the code to use a do...while loop.
var secret;
var correctGuess = false;
do {
var secret = prompt("What is the secret password?");
if (secret === 'sesame') {
correctGuess = true;
}
} while ( !correctGuess )
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer

brandon downs
11,577 PointsYou are really close!
I believe you are running into a scoping issue. you declared the variable secrets in the global scope, and then you also declare the variable secrets in the scope of the do... while loop.
var secret;
var correctGuess = false;
do {
var secret = prompt("What is the secret password?");
if (secret === 'sesame') {
correctGuess = true;
}
} while ( !correctGuess )
if you get rid of the var declaration inside the do.. while loop it works fine!
var secret;
var correctGuess = false;
do {
secret = prompt("What is the secret password?");
if (secret === 'sesame') {
correctGuess = true;
}
} while ( !correctGuess )
you can also get away without creating the variable correctGuess
var secret;
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" )
I hope this helps!
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsModerator Note:
Duplicate post have been deleted from the Community for this question. Please refrain from posting multiple threads for the same question.
Thank-you :)
Jason