Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial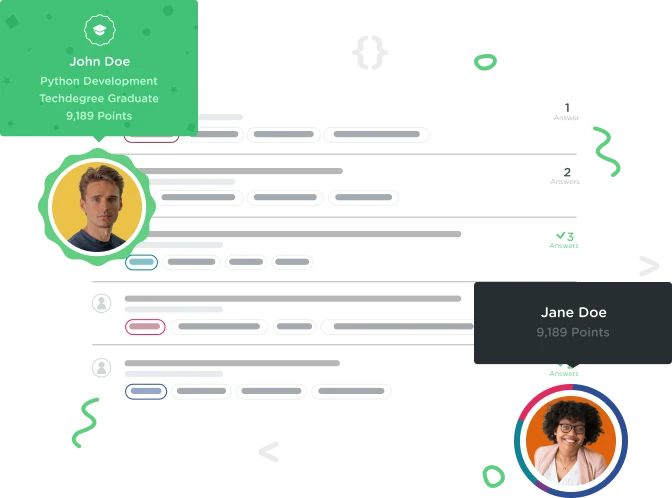

Adam Fields
4,487 PointsCreate a for loop that logs the numbers 4 to 156 to the console. To log a value to the console use the console.log( )
what am I doing wrong?
var num;
for(var i=4; i<=156;+=1)
num+=1 + i;
console.log(num);
}
2 Answers
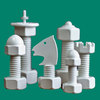
Steven Parker
231,269 PointsI don't understand those calculations with "num", but you really don't need "num" at all. Just log the loop variable "i".
But you also have a syntax error in the final "for" loop clause: instead of "+=1
" you need "i+=1
" (to increment "i").
I'll bet you can get it now without an explicit code spoiler.

Kieran Barker
15,028 PointsHey Adam,
You're close! This is the correct code for this challenge:
for ( var i = 4; i <= 156; i++ ) {
console.log( i );
}
What this code is doing is as follows:
- Initializing a counter variable with the value
4
. - Before each loop iteration, the JavaScript interpreter checks to see if the current value of
i
(which will be4
the first time around) is less than156
. If it is, then the loop will run. - The JavaScript interpreter executes everything inside the code block β thatβs everything inside the curly braces. In this case, the current value of
i
is logged to the console each time the loop runs. - At the end of the loop, the counter variable --
i
-- is updated by1
. So the next time around it will be5
. The loop will stop running when the condition is no longer true, i.e. wheni
is no longer less than156
.
A quick note...
- I used
++
to increcementi
, buti += 1
will do the same thing.
Please let me know if you have any questions!
~ Kieran
Adam Fields
4,487 PointsAdam Fields
4,487 Pointsthank you steven I figured it out thank you .