Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial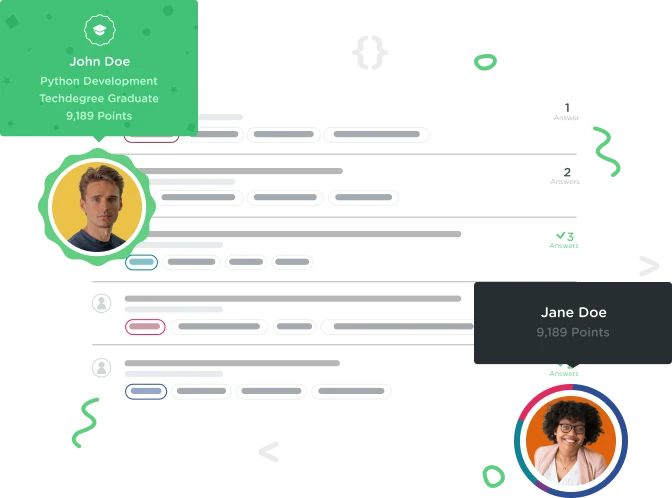
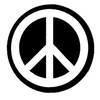
john larson
16,594 PointsCreate a function named combo() that takes two iterables and returns a list of tuples: this code works but..
def combo(iter1, iter2):
output = []
for index, value in enumerate(iter1):
# I don't see where [index] is defined for iter2
# I suspect that iterating over iter2 is happening
# just being inside the for loop
# is that where [index] is coming from also?
# by some association with iter1, being inside the loop?
output.append((value, iter2[index]))
return output
x = combo([1, 2, 3], 'abc')
print(x)
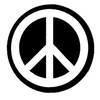
john larson
16,594 PointsCheo, you gave a beautiful in-depth explanation that I will go through in detail. I think you answered my question right off. Are you saying that the index that is referenced here:
- output.append((value, iter2[index]))
- is actually the index from iter1
- and that number is used to collaberate the two iterables?
- cause if it is, that is very clever, and I wouldn't have thought of it on my own
Cheo R
37,150 PointsCheo R
37,150 PointsRemember that values can be retrieved by getting the index of a list.
Your code:
Is making a tuple of two values:
Say the first list is longer than the second list:
What do you think will happen?
It gives and IndexError because the second list does not have a value at that index.