Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial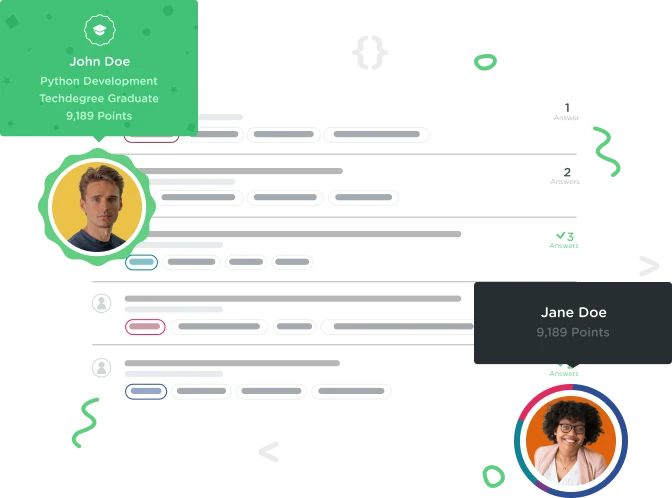
Chris Grazioli
31,225 PointsCreate a function named combo that takes two ordered iterables.
I'm having trouble working through this problem, is this a packing or unpacking deal? I'm pretty sure python has a zip() function for something like this, but I think for lists and no in the spirit of this exercise.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(a,b):
list_of=[]
for item1, item2 in a, b:
list_of.append(tuple([item1,item2]))
return list_of
2 Answers
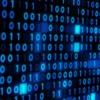
Alexander Davison
65,469 PointsUnfortunately you aren't allow to do this kind of thing in Python:
a = [1,2,3]
b = [1,2,3]
# The loop below is not valid Python
for x, y in a, b:
pass
So, you'll have to manually remember the index:
def combo(a,b):
result = []
for x in range(len(a)):
result.append( (a[x], b[x]) )
return result
I hope this helps
If you have any questions, feel free to ask!
Happy C0D1NG!
~Alex
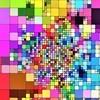
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthat's also what i did. there's sort of a clue to use indices when it says the iterables will be the same length. also, this particular challenge is throwing an error if you try to use zip, they want you to cook up your own solution. but just for fun here is a one-liner with zip!
def combo(a,b):
return list(zip(a,b))
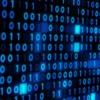
Alexander Davison
65,469 PointsActually the code challenge doesn't allow usages of the zip()
function.